Conventional Beamformer¶
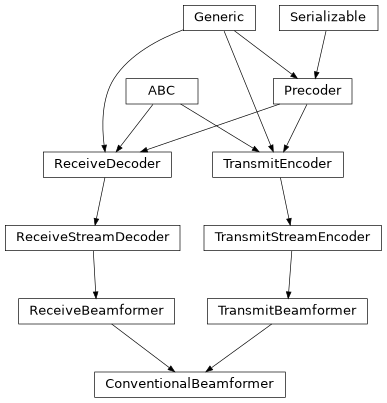
Also refferred to as delay and sum beamformer.
The Bartlett[1] beamformer, also known as conventional or delay and sum beamformer, maximizes the power transmitted or received towards a single direction of interest \((\theta, \phi)\), where \(\theta\) is the zenith and \(\phi\) is the azimuth angle of interest in spherical coordinates, respectively.
Let \(\mathbf{X} \in \mathbb{C}^{N \times T}\) be the the matrix of \(T\) time-discrete samples acquired by an antenna arrary featuring \(N\) antennas. The antenna array’s response towards a source within its far field emitting a signal of small relative bandwidth is \(\mathbf{a}(\theta, \phi) \in \mathbb{C}^{N}\). Then
is the Conventional beamformer’s power estimate with
being the beamforming weights to steer the sensor array’s receive characteristics towards direction \((\theta, \phi)\), so that
is the implemented beamforming equation.
The following section provides an example for the usage of the Conventional Beamformer in a communication scenario.
For this a new simulation environment is initialised. A digital antenna array consisting of ideal isotropic antenna elements spaced at half wavelength intervals is created and is assigned to a device representing a base station.
1# Initialize a new simulation
2from hermespy.simulation import Simulation
3simulation = Simulation(seed=42)
4
5# Create a new device and assign it the antenna array
6from hermespy.simulation import SimulatedIdealAntenna, SimulatedUniformArray
7from hermespy.core import Transformation
8import numpy as np
9
10base_station_device = simulation.new_device(
11 antennas=SimulatedUniformArray(SimulatedIdealAntenna, .5 * wavelength, (4, 4, 1)),
12 carrier_frequency=carrier_frequency,
13 pose=Transformation.From_Translation(np.array([0, 0, 0])),
14)
To probe the characterestics a cosine waveform is generated as the transmit waveform.
1# Configure a probing signal to be transmitted from the base station
2from hermespy.modem import RootRaisedCosineWaveform, SingleCarrierLeastSquaresChannelEstimation, SingleCarrierZeroForcingChannelEqualization, SingleCarrierCorrelationSynchronization
3
4waveform = RootRaisedCosineWaveform(
5 symbol_rate=sampling_rate//2,
6 oversampling_factor=2,
7 num_preamble_symbols=32,
8 num_data_symbols=128,
9 roll_off=.9,
10)
11waveform.synchronization = SingleCarrierCorrelationSynchronization()
12waveform.channel_estimation = SingleCarrierLeastSquaresChannelEstimation()
13waveform.channel_equalization = SingleCarrierZeroForcingChannelEqualization()
The base station device is configured to transmit the beamformed signal by assigning the Conventional Beamformer to the base station device.
1# Configure the base station device to transmit the beamformed probing signal
2from hermespy.beamforming import ConventionalBeamformer
3from hermespy.modem import TransmittingModem
4from copy import deepcopy
5
6beamformer = ConventionalBeamformer()
7base_station_device.transmit_coding[0] = beamformer
8
9base_station_transmitter = TransmittingModem(waveform=deepcopy(waveform))
10base_station_device.add_dsp(base_station_transmitter)
Now the simulation can be extended to evalaute the performance in a real world communication scenario. For this two devices representing the UEs are added, to be illuminated by the BS.
1# Create Two simulated device representing the user equipments
2user_equipment_device_1 = simulation.new_device(
3 carrier_frequency=carrier_frequency,
4 pose=Transformation.From_Translation(np.array([100., 100., 100.])),
5)
6user_equipment_device_2 = simulation.new_device(
7 carrier_frequency=carrier_frequency,
8 pose=Transformation.From_Translation(np.array([100., -100., 100.])),
9)
The user equipments can be configured to receive the signal by setting them up as receiving modems.
1# Configure the user equipments to receive the signal
2from hermespy.modem import ReceivingModem
3
4user_equipment_receiver_1 = ReceivingModem(waveform=deepcopy(waveform))
5user_equipment_receiver_2 = ReceivingModem(waveform=deepcopy(waveform))
6user_equipment_device_1.add_dsp(user_equipment_receiver_1)
7user_equipment_device_2.add_dsp(user_equipment_receiver_2)
Now as defined the Conventional Beamformer illuminates the maximum radiation on one UE. This can be realised by configuring the transmit foucs of the Beamformer.
1# Focus the base station's main lobe on the desired user equipment and nulls on the others
2from hermespy.simulation import DeviceFocus
3
4beamformer.transmit_focus = [
5 DeviceFocus(user_equipment_device_1), # Focus on User Equipmment 1
6]
The propgation characterestics between the BS and the UEs can be modelled using the SpatialDelayChannel Model.
1# Configure a channel between base station and the UEs
2from hermespy.channel import SpatialDelayChannel
3
4simulation.set_channel(
5 base_station_device,
6 user_equipment_device_1,
7 SpatialDelayChannel(model_propagation_loss=False),
8)
9
10simulation.set_channel(
11 base_station_device,
12 user_equipment_device_2,
13 SpatialDelayChannel(model_propagation_loss=False),
14)
Now hermespy can be instructed to conduct a simulation campaign to evaluate the received signal power at the UEs by adding the evaluator.
1# Run the simulation and the evaluate the received power from the respective UEs.
2from hermespy.core import ReceivePowerEvaluator
3
4simulation.add_evaluator(ReceivePowerEvaluator(user_equipment_receiver_1))
5simulation.add_evaluator(ReceivePowerEvaluator(user_equipment_receiver_2))
6
7# Creating a new dimension to dynamically switch the focus of the beamformer during the simulation campaign.
8simulation.new_dimension(
9 'focused_device',
10 [user_equipment_device_1, user_equipment_device_2],
11 beamformer.transmit_focus[0]
12)
13
14result = simulation.run()
In the previous example the Conventional Beamformer was assigned to transmitting base station. The same example can be adpated to study the performance of the beamformer assigned to a receiving base station.
The base station is configured for reception.
1# Configure the base station device to receive the beamformed probing signal
2from hermespy.beamforming import ConventionalBeamformer
3from hermespy.modem import ReceivingModem
4from copy import deepcopy
5
6beamformer = ConventionalBeamformer()
7base_station_device.receive_coding[0] = beamformer
8
9base_station_receiver = ReceivingModem(waveform=deepcopy(waveform))
10base_station_device.add_dsp(base_station_receiver)
The UEs will be configured as transmitting.
1# Configure the user equipments to transmit the signal
2from hermespy.modem import TransmittingModem
3
4user_equipment_transmitter_1 = TransmittingModem(waveform=deepcopy(waveform))
5user_equipment_transmitter_2 = TransmittingModem(waveform=deepcopy(waveform))
6user_equipment_device_1.add_dsp(user_equipment_transmitter_1)
7user_equipment_device_2.add_dsp(user_equipment_transmitter_2)
The receieve focus of the beamformer will be configured.
1# Focus the base station's main lobe on the desired user equipment.
2from hermespy.simulation import DeviceFocus
3
4beamformer.receive_focus = [
5 DeviceFocus(user_equipment_device_1), # Focus on User Equipmment 1
6]
The performance of the beamformer is studied by analysing the received signal quality from the respective UEs. For this purpose, the Error Vector Magnitude of the consetallation diagram of the Received signal is evaluated.
1# Run the simulation and the inspect the received signal quality from the respective UEs.
2from hermespy.modem import ConstellationEVM
3
4simulation.add_evaluator(ConstellationEVM(user_equipment_transmitter_1, base_station_receiver))
5simulation.add_evaluator(ConstellationEVM(user_equipment_transmitter_2, base_station_receiver))
6
7
8# Creating a new dimension to dynamically switch the focus of the beamformer during the simulation campaign.
9simulation.new_dimension(
10 'focused_device',
11 [user_equipment_device_1, user_equipment_device_2],
12 beamformer.receive_focus[0]
13)
14
15result = simulation.run()
- class ConventionalBeamformer[source]¶
Bases:
TransmitBeamformer
,ReceiveBeamformer
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- _decode(samples, carrier_frequency, angles, array)[source]¶
Decode signal streams for receive beamforming.
This method is called as a subroutine during
decode_streams
andprobe
.- Parameters:
samples (
ndarray
) – Signal samples, first dimension being the number of signal streams \(N\), second the number of samples \(T\).carrier_frequency (
float
) – The assumed carrier central frequency of the samples \(f_\mathrm{c}\).angles (
ndarray
) – Spherical coordinate system angles of arrival in radians. A three-dimensional numpy array with the first dimension representing the number of angles, the second dimension of magnitude number of focus points \(F\), and the third dimension containing the azimuth and zenith angle in radians, respectively.array (
AntennaArrayState
) – The assumed antenna array.
- Return type:
- Returns:
Stream samples of the focused signal towards all focus points. A three-dimensional numpy array with the first dimension representing the number of focus points, the second dimension the number of returned streams and the third dimension the amount of samples.
- _encode(samples, carrier_frequency, focus_angles, array)[source]¶
Encode signal streams for transmit beamforming.
- Parameters:
samples (
ndarray
) – Signal samples, first dimension being the number of transmit antennas, second the number of samples.carrier_frequency (
float
) – The assumed central carrier frequency of the samples generated RF signal after mixing in Hz.focus_angles (
ndarray
) – Focused angles of departure in radians. Two-dimensional numpy array with the first dimension representing the number of focus points and the second dimension of magnitude two being the azimuth and elevation angles, respectively.array (
AntennaArrayState
) – The assumed antenna array.
- Return type:
- Returns:
The encoded signal samples. Two-dimensional complex-valued numpy array with the first dimension representing the number of transmit antennas streams and the second dimension the number of samples.
- num_receive_output_streams(num_input_streams)[source]¶
Get required number of output streams during decoding.
Returns: The number of output streams. Negative numbers indicate infeasible configurations.
- num_transmit_input_streams(num_output_streams)[source]¶
Get required number of input streams during encoding.
Returns: The number of input streams. Negative numbers indicate infeasible configurations.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- property num_receive_focus_points: int¶
Number of required receive focus points.
If this is \(1\), the beamformer is considered to be a single focus point beamformer and
receive_focus
will return a single focus point. Otherwise, the beamformer is considered a multi focus point beamformer andreceive_focus
will return aSequence
of focus points.Returns: Number of focus points.
- property num_transmit_focus_points: int¶
Number of required transmit focus points.
If this is \(1\), the beamformer is considered to be a single focus point beamformer and
transmit_focus
will return a single focus point. Otherwise, the beamformer is considered a multi focus point beamformer andtransmit_focus
will return aSequence
of focus points.