Mapping¶
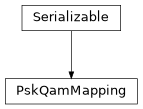
Mapping between bits and PSK/QAM/PAM constellation This module provides a class for a PSK/QAM mapper/demapper.
The following features are supported:
arbitrary 2D (complex) constellation mapping can be given
default Gray-coded constellations for BPSK, QPSK, 8-PSK, 4-, 8-, 16- PAM, 16-, 64- and 256-QAM are provided
all default constellations follow 3GPP standards TS 36.211 (except 8-PSK, which is not defined in 3GPP)
hard and soft (LLR) output are available
This implementation has currently the following limitations:
LLR available only for default BPSK, QPSK, 4-, 8-, 16- PAM, 16-, 64- and 256-QAM
only linear approximation of LLR is considered, similar to the one described in:
Tosato, Bisaglia, “Simplified Soft-Output Demapper for Binary Interleaved COFDM with Application to HIPERLAN/2”, Proceedings of IEEE International Commun. Conf. (ICC) 2002
- class PskQamMapping(modulation_order, mapping=None, soft_output=False, is_complex=True)[source]¶
Bases:
Serializable
Implements the mapping of bits into complex numbers, following a PSK/QAM modulation.
- modulation_order¶
size of modulation constellation.
- bits_per_symbol¶
number of bits in modulation symbol.
- soft_output¶
if True, then soft output (LLR) will be provided. # If False, then estimated bits are returned.
- Parameters:
modulation_order (
int
) – Number of points in the constellation. Must be a power of two.mapping (
ndarray
|None
) – Vector with length modulation_order defining the mapping between bits and modulation symbols. At each symbol, bits are input MSB first. For example, with a 32-point constellation, the bit sequence 01101 corresponds to the decimal 13, and hence will be mapped to the 13-th element in ‘mapping’ vector. It is optional for certain modulation orders, which are given in PskQamMapping.mapping_available, and for which a default mapping is provided.soft_output (
bool
) – if True, then soft output (LLR) will be provided. If False, then estimated bits (0 or 1) are returned.is_complex (
bool
) – if True, then complex modulation is considered (PSK/QAM), if False, then real-valued modulation is considered (PAM)
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- detect_bits(rx_symbols, noise_variance=1.0)[source]¶
Returns either bits or LLR for the provided symbols.
- Parameters:
rx_symbols (numpy.ndarray) – Vector of N received symbols, for which the bits/LLR will be estimated
noise_variance (
ndarray
|float
) – vector with the noise variance in each received symbol. If a scalar is given, then the same variance is assumed for all symbols. This is only relevant if ‘self.soft_output’ is true.
- Return type:
- Returns:
Vector of N * self.bits_per_symbol elements containing either the estimated bits or the LLR values of each bit, depending on the value of ‘self.soft_output’
- static generate_pam_symbol_3gpp(modulation_order, bits)[source]¶
Returns 1D amplitudes following 3GPP modulation mapping.
3GPP has defined in TS 36.211 mapping tables from bits into complex symbols. Since the mapping from bits into amplitudes is the same for both I and Q components, and this function maps blocks of N bits into one of M=2^N possible (real-valued) amplitudes.
- Parameters:
- Return type:
- Returns:
Vector of K real-valued symbols. Note that the symbols are not normalized, and range from -(M-1) to (M+1) with step 2, e..g., for M=8, values can be -7, -5, -3, -1, 1, 3, 5, 7.
- static get_llr_3gpp(modulation_order, rx_symbols, noise_variance, is_complex)[source]¶
Returns LLR for each bit based on a received symbol, following 1D 3GPP modulation mapping.
3GPP has defined in TS 36.211 mapping tables from bits into complex symbols. Since the mapping from bits into amplitudes is the same for both I and Q components, and this function maps received real-valued amplitudes into blocks of N = log2(M) log-likelihood ratios (LLR) for all bits, with M the modulation order.
Only linear approximation of LLR is considered, similar to the one described in: Tosato, Bisaglia, “Simplified Soft-Output Demapper for Binary Interleaved COFDM with Application to HIPERLAN/2”, Proceedings of IEEE International Commun. Conf. (ICC) 2002
LLR calculation is available for real-valued modulations of order 2, 4, 8 or 16.
LLR is returned considering unit-power Gaussian noise at all symbols.
- Parameters:
modulation_order (
int
) – modulation order M. M=2, 4, 8, 16 are supportedrx_symbols (
ndarray
) – array with K received symbolsnoise_variance (
ndarray
) – float or array with noise varaiance of K symbolsis_complex (
bool
) – if True, then complex modulation is considered (PSK/QAM), If False, then real-valued modulation is considered (PAM)
- Return type:
Returns: Vector of N x K elements with the LLR values.
- get_mapping()[source]¶
Returns current mapping table
- Return type:
- Returns:
array with M modulation_order elements containing all possible modulation symbols. See specifications in “PskQamMapping.__init__”
- get_symbols(bits)[source]¶
Calculates the complex numbers corresponding to the information in ‘bits’.
Note
The constellation is normalized, such that the mean symbol energy is unitary.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- mapping_available = [2, 4, 8, 16, 64, 64, 256]¶
List of available default constellations.
- mapping_available_pam = [2, 4, 8, 16]¶
List of available default constellations for PAM.