Discrete Fourier Transform¶
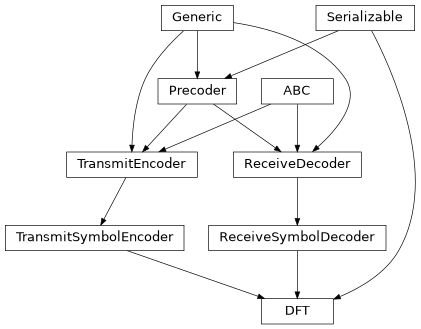
Discrete Fourier precodings apply a Fourier transform to the input symbols in between the mapping and modulation stage during transmission. Inversely, the precoding is applied after demodulation and before the demapping stage during reception.
They can be configured by adding an instance to the precoding configuration
of a Modem
or Modem
exposed by the TransmittingModemBase.precoding
/ ReceivingModemBase.precoding
attributes:
1# Create a new simulation featuring a single device
2simulation = Simulation()
3device = simulation.new_device()
4
5# Create a new modem
6# This should be replaced by a BaseModem implementation such as DuplexLink
7modem = BaseModem()
8device.add_dsp(modem)
9
10# Configure an OFDM waveform
11modem.waveform = OFDMWaveform(
12 oversampling_factor=2,
13 num_subcarriers=1024,
14 grid_resources=[GridResource(
15 repetitions=100,
16 prefix_ratio=0.0684,
17 elements=[
18 GridElement(ElementType.DATA, 9),
19 GridElement(ElementType.REFERENCE, 1),
20 ]
21 )],
22 grid_structure=[SymbolSection(3, [0])]
23)
24
25# Configure the precoding
26modem.transmit_symbol_coding[0] = DFT()
- class DFT(fft_norm=DFTNorm.ORTHO)[source]¶
Bases:
TransmitSymbolEncoder
,ReceiveSymbolDecoder
,Serializable
A precoder applying the Discrete Fourier Transform to each data stream.
- Parameters:
fft_norm (
DFTNorm
) – The norm applied to the discrete fourier transform. Defaults to DFTNorm.ORTHO. See also numpy.fft.fft for details
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- decode_symbols(symbols, num_output_streams)[source]¶
Decode a data stream before reception.
This operation may modify the number of streams as well as the number of data symbols per stream.
- Parameters:
symbols (StatedSymbols) – Symbols to be decoded.
num_output_streams (int) – Number of required output streams after decoding.
- Return type:
Returns: Decoded symbols.
- encode_symbols(symbols, num_output_streams)[source]¶
Encode a data stream before transmission.
This operation may modify the number of streams as well as the number of data symbols per stream.
- Parameters:
symbols (StatedSymbols) – Symbols to be encoded.
num_output_streams (int) – Number of required output streams after encoding.
- Return type:
Returns: Encoded symbols.
- num_receive_output_streams(num_input_streams)[source]¶
Get required number of output streams during decoding.
Returns: The number of output streams. Negative numbers indicate infeasible configurations.
- num_transmit_input_streams(num_output_streams)[source]¶
Get required number of input streams during encoding.
Returns: The number of input streams. Negative numbers indicate infeasible configurations.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type: