Frame Generation¶
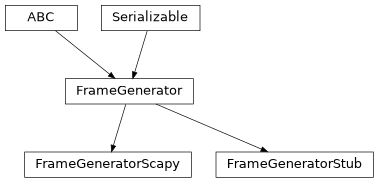
- class FrameGenerator[source]¶
Bases:
ABC
,Serializable
Base class for frame generators.
- abstract pack_frame(source, num_bits)[source]¶
Generate a frame of num_bits bits from the given bitsource.
- Parameters:
source (
BitsSource
) – Payload source.num_bits (
int
) – Number of bits in the whole resulting frame.
- Return type:
Returns: Array of ints with each element beeing an individual bit.
- class FrameGeneratorStub[source]¶
Bases:
FrameGenerator
A dummy placeholder frame generator, packing and unpacking payload without any overhead.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- pack_frame(source, num_bits)[source]¶
Generate a frame of num_bits bits from the given bitsource.
- Parameters:
source (
BitsSource
) – Payload source.num_bits (
int
) – Number of bits in the whole resulting frame.
- Return type:
Returns: Array of ints with each element beeing an individual bit.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- class FrameGeneratorScapy(packet)[source]¶
Bases:
FrameGenerator
Scapy wrapper frame generator.
- Attrs:
packet: Scapy packet header to which a payload would be attached. packet_type: Type of the first layer of the packet header.
- Parameters:
packet (
Packet
) – Packet to which a payload will be attached.
- pack_frame(source, num_bits)[source]¶
Generate a frame of num_bits bits from the given bitsource. Note that the payload size is num_bits minus number of bits in the packet header. Note that payload can be of size 0, in which case no data would be sent (except for the packet header).
- Parameters:
source (
BitsSource
) – Payload source.num_bits (
int
) – Number of bits in the whole resulting frame.
- Raises:
ValueError – If num_bits is not enough to fit the packet.
- Return type: