Single Target¶
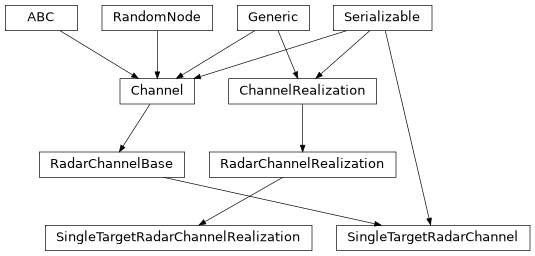
Model of a radar channel featuring a single reflecting target.
The following minimal example outlines how to configure the channel model
within the context of a Simulation
:
1# Initialize a single device operating at 78.5 GHz
2simulation = Simulation(seed=42)
3device = simulation.new_device(carrier_frequency=78.5e9)
4
5# Create a radar channel modeling a single target at 10m distance
6channel = SingleTargetRadarChannel(10, 1, attenuate=False)
7simulation.set_channel(device, device, channel)
8
9# Configure an FMCW radar with 5 GHz bandwidth illuminating the target
10radar = Radar(FMCW(10, 5e9, 90 / 5e9, 100 / 5e9))
11device.add_dsp(radar)
12
13# Configure a simulation evluating the radar's operating characteristics
14simulation.add_evaluator(ReceiverOperatingCharacteristic(radar, device, device, channel))
15simulation.new_dimension('noise_level', dB(np.arange(0, -22, -2).tolist()), device)
16simulation.num_samples = 1000
17
18# Run simulation and plot resulting ROC curve
19result = simulation.run()
20result.plot()
- class SingleTargetRadarChannel(target_range, radar_cross_section, target_azimuth=0.0, target_zenith=0.0, target_exists=True, velocity=0.0, decorrelation_distance=inf, attenuate=True, gain=1.0, seed=None)[source]¶
Bases:
RadarChannelBase
[SingleTargetRadarChannelRealization
],Serializable
Model of a radar channel featuring a single reflecting target.
- Parameters:
target_range (
Union
[float
,Tuple
[float
,float
]]) – Absolute distance of target and radar sensor in meters. Either a specific distance or a range of minimal and maximal target distance.radar_cross_section (
float
) – Radar cross section (RCS) of the assumed single-point reflector in m**2target_azimuth (
Union
[float
,Tuple
[float
,float
]]) – Target location azimuth angle in radians, considering spherical coordinates. Zero by default.target_zenith (
Union
[float
,Tuple
[float
,float
]]) – Target location zenith angle in radians, considering spherical coordinates. Zero by default.target_exists (
bool
) – True if a target exists, False if there is only noise/clutter (default 0 True)velocity (
Union
[float
,Tuple
[float
,float
],ndarray
]) – Velocity as a 3D vector (or as a float), in m/s (default = 0)decorrelation_distance (
float
) – Distance at which channel samples are considered to be uncorrelated. \(\infty\) by default, i.e. the channel is considered to be fully correlated in space.attenuate (
bool
) – If True, then signal will be attenuated depending on the range, RCS and losses. If False, then received power is equal to transmit power.
- Raises:
ValueError – If radar_cross_section < 0. If carrier_frequency <= 0. If more than one antenna is considered.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- _realize()[source]¶
Generate a new channel realzation.
Abstract subroutine of
realize
. EachChannel
is required to implement their own_realize()
method.Returns: A new channel realization.
- Return type:
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- property decorrelation_distance: float¶
Decorrelation distance of the channel.
- Raises:
ValueError – If the decorrelation distance is smaller than zero.
- property radar_cross_section: float¶
Access configured radar cross section.
- Returns:
radar cross section [m**2]
- Return type:
- property target_azimuth: float | Tuple[float, float]¶
Target position azimuth in spherical coordiantes.
- Returns:
Azimuth angle in radians.
- property target_range: float | Tuple[float, float]¶
Absolute distance of target and radar sensor.
Returns: Target range in meters.
- Raises:
ValueError – If the range is smaller than zero.
- class SingleTargetRadarChannelRealization(consistent_realization, target_range_variable, target_azimuth_variable, target_zenith_variable, target_velocity_variable, target_phase_variable, target_range, target_azimuth, target_zenith, target_cross_section, target_velocity, attenuate, sample_hooks, gain)[source]¶
Bases:
RadarChannelRealization
Realization of a single target radar channel.
Generated by realizing a
SingleTargetRadarChannel
.- Parameters:
gain (
float
) – Linear power gain factor a signal experiences when being propagated over this realization.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type: