Ideal Channel¶
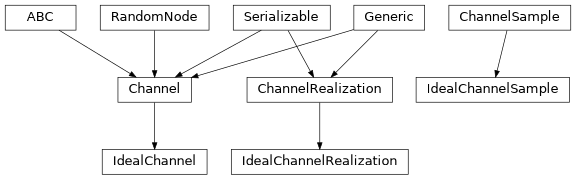
The IdealChannel
is the default Channel
assumed by Simulations
.
It is completely deterministic and lossless, introducing neither phase shifts, amplitude changes nor propagation delays
in between the linked Devices
.
classDiagram direction LR class IdealChannel { _realize() : IdealChannelRealization } class IdealChannelRealization { _sample() : IdealChannelSample } class IdealChannelSample { propagate(Signal) : Signal } IdealChannel --o IdealChannelRealization : realize() IdealChannelRealization --o IdealChannelSample : sample() click IdealChannel href "#hermespy.channel.ideal.IdealChannel" click IdealChannelRealization href "#hermespy.channel.ideal.IdealChannelRealization" click IdealChannelSample href "#hermespy.channel.ideal.IdealChannelSample"
Considering two devices \(\alpha\) and \(\beta\) featuring \(N_\alpha\) and \(N_\beta\) antennas respectively, the ideal Channel’s impulse response
depends on the number of antennas of the devices and is independent of the time \(t\). For channels with an unequal number of antennas, the ideal Channel’s impulse response is a diagonal matrix with ones on the diagonal, padded with zeros to match the dimensions of the channel matrix. Therefore, the device with the bigger amount of antennas will receive / transmit nothing from the additional antennas.
- class IdealChannel(gain=1.0, seed=None)[source]¶
Bases:
Channel
[IdealChannelRealization
,IdealChannelSample
]An ideal distortion-less channel model.
- Parameters:
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- _realize()[source]¶
Generate a new channel realzation.
Abstract subroutine of
realize
. EachChannel
is required to implement their own_realize()
method.Returns: A new channel realization.
- Return type:
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- class IdealChannelRealization(sample_hooks=None, gain=1.0)[source]¶
Bases:
ChannelRealization
[IdealChannelSample
]Realization of an ideal channel.
Generated by the
_realize()
routine ofIdealChannels
.- Parameters:
sample_hooks (
Optional
[Set
[ChannelSampleHook
[TypeVar
(CST
, bound= ChannelSample)]]]) – Hooks to be called after the channel is sampled.gain (
float
) – Linear power gain factor a signal experiences when being propagated over this realization. \(1.0\) by default, meaning no gain or loss.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- _sample(state)[source]¶
Sample the channel realization at a given point in time and space.
Abstract subroutine of
sample
.- Parameters:
state (
LinkState
) – State of the channel at the time of sampling.- Return type:
Returns: The channel sample at the given point in time.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- class IdealChannelSample(gain, state)[source]¶
Bases:
ChannelSample
Sample of an ideal channel realization.
Generated by the
_sample
routine ofIdealChannelRealization
.- Parameters:
- _propagate(signal, interpolation)[source]¶
Propagate radio-frequency band signals over a channel instance.
Abstract subroutine of
propagate
.- Parameters:
signal (
SignalBlock
) – The signal block to be propagated.interpolation (
InterpolationMode
) – Interpolation behaviour of the channel realization’s delay components with respect to the proagated signal’s sampling rate.
- Return type:
Returns: The propagated signal.
- state(num_samples, max_num_taps, interpolation_mode=InterpolationMode.NEAREST)[source]¶
Generate the discrete channel state information from this channel realization.
Denoted by
\[\mathbf{H}^{(m, \tau)} \in \mathbb{C}^{N_{\mathrm{Rx}} \times N_{\mathrm{Tx}}}\]within the respective equations.
- Parameters:
num_samples (
int
) – Number of discrete time-domain samples of the chanel state information.max_num_taps (
int
) – Maximum number of delay taps considered per discrete time-domain sample.interpolation_mode (
InterpolationMode
) – Interpolation behaviour of the channel realization’s delay components with respect to the proagated signal’s sampling rate. If not specified, an integer rounding to the nearest sampling instance will be assumed.
- Return type:
Returns: The channel state information representing this channel realization.