Receiving Modem¶
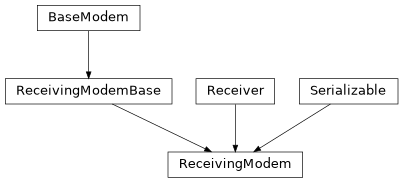
Receiving modems represent the digital signal processing operations performed within a communication system for the point of analog-to-digital conversion up to the point of decoding the received bits.
After a ReceivingModem
is added as a
type of Receiver
to a Device
,
a call to Device.receive
will be delegated
to ReceivingModem._receive
:
sequenceDiagram Device ->>+ ReceivingModem: receive(Signal) ReceivingModem->>+CommunicationWaveform: synchronize(Signal) CommunicationWaveform->>-ReceivingModem: frame_indices loop Frame Reception ReceivingModem->>+ReceiveStreamCoding: decode(Signal) ReceiveStreamCoding->>-ReceivingModem: Signal ReceivingModem->>+CommunicationWaveform: demodulate(Signal) CommunicationWaveform->>-ReceivingModem: Symbols ReceivingModem->>+CommunicationWaveform: estimate_channel(Symbols) CommunicationWaveform->>-ReceivingModem: StatedSymbols ReceivingModem->>+CommunicationWaveform: pick(StatedSymbols) CommunicationWaveform->>-ReceivingModem: StatedSymbols ReceivingModem->>+SymbolPrecoding: decode(StatedSymbols) SymbolPrecoding->>-ReceivingModem: StatedSymbols ReceivingModem->>+CommunicationWaveform: equalize_symbols(StatedSymbols) CommunicationWaveform->>-ReceivingModem: Symbols ReceivingModem->>+CommunicationWaveform: unmap(StatedSymbols) CommunicationWaveform->>-ReceivingModem: Bits ReceivingModem->>+EncoderManager: decode(Bits) EncoderManager->>-ReceivingModem: Bits end ReceivingModem ->>- Device: CommunicationReception
Initially, the ReceivingModem
will synchronize incoming
Signals
, partitionin them into individual frames.
For each frame, the ReceiveSignalCoding
configured by the receive_signal_coding
will be used to decode the incoming base-band sample streams from each AntennaPort
.
Afterwards, each decoded stream will be demodulated
,
the channel will be estimated
and
the resulting StatedSymbols
will be picked
.
The StatedSymbols
will then be decoded
and equalized
.
Finally, the Symbols
will be unmapped
and the error correction will be decoded
.
Note that, as a bare minimum, only the waveform
has to be configured for a fully functional ReceivingModem
.
The following snippet shows how to configure a ReceivingModem
with a RootRaisedCosineWaveform
wavform implementing a
CommunicationWaveform
:
1# Initialize a new simulation considering a single device
2simulation = Simulation()
3device = simulation.new_device(carrier_frequency=1e10)
4
5# Configure the modem modeling the device's transmit DSP
6rx_modem = ReceivingModem()
7device.receivers.add(rx_modem)
8
9# Configure the modem's waveform
10waveform = RootRaisedCosineWaveform(
11 oversampling_factor=4,
12 symbol_rate=1e6,
13 num_preamble_symbols=16,
14 num_data_symbols=32,
15 modulation_order=64,
16)
The barebone configuration can be extend by additional components such as
Synchronization
,
ReceiveSignalCoding
,
Channel Estimation
,
ReceiveSymbolCoding
,
Channel Equalization
and
Bit Encoders
:
1
2# Configure the waveform's synchronization routine
3rx_modem.waveform.synchronization = SingleCarrierCorrelationSynchronization()
4
5# Add a custom stream precoding to the modem
6rx_modem.receive_signal_coding[0] = ConventionalBeamformer()
7
8# Add a custom symbol precoding to the modem
9rx_modem.receive_symbol_coding[0] = DFT()
10
11# Configure the waveform's channel estimation routine
12rx_modem.waveform.channel_estimation = SingleCarrierLeastSquaresChannelEstimation()
13
14# Configure the waveform's channel equalization routine
15rx_modem.waveform.channel_equalization = SingleCarrierZeroForcingChannelEqualization()
16
17# Add forward error correction encodings to the transmitted bit stream
18rx_modem.encoder_manager.add_encoder(RepetitionEncoder(32, 3))
19rx_modem.encoder_manager.add_encoder(BlockInterleaver(192, 32))
20
21# Generate a transmission to be received by the modem
- class ReceivingModem(selected_receive_ports=None, carrier_frequency=None, receive_symbol_coding=None, receive_signal_coding=None, encoding=None, waveform=None, frame_generator=None, seed=None)[source]¶
Bases:
ReceivingModemBase
[CommunicationWaveform
],Receiver
[CommunicationReception
],Serializable
Representation of a wireless modem exclusively receiving.
- Parameters:
selected_receive_ports (
Optional
[Sequence
[int
]]) – Indices of antenna ports selected for reception from the operatedDevice's
antenna array. If not specified, all available antenna ports will be considered.carrier_frequency (
float
|None
) – Carrier frequency of the received communication signal.receive_symbol_coding (
Union
[ReceiveSymbolCoding
,Sequence
[ReceiveSymbolDecoder
],None
]) – Complex communication symbol coding configuration during reception.receive_signal_coding (
Union
[ReceiveSignalCoding
,Sequence
[ReceiveStreamDecoder
],None
]) – Stream MIMO coding configuration during signal reception.encoding (
Union
[EncoderManager
,Sequence
[Encoder
],None
]) – Bit coding configuration.waveform (
CommunicationWaveform
|None
) – The waveform to be received by this modem.frame_generator (
FrameGenerator
|None
) – Frame generator used to pack and unpack communication frames. If not specified, a stub generator will be assumed.seed (
int
|None
) – Seed used to initialize the pseudo-random number generator.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- class ReceivingModemBase(receive_symbol_coding=None, receive_signal_coding=None, encoding=None, waveform=None, frame_generator=None, seed=None)[source]¶
Bases:
Generic
[CWT
],BaseModem
[CWT
]Base class of signal processing algorithms receiving information.
- Parameters:
receive_symbol_coding (
Union
[ReceiveSymbolCoding
,Sequence
[ReceiveSymbolDecoder
],None
]) – Complex communication symbol coding configuration during reception.receive_signal_coding (
Union
[ReceiveSignalCoding
,Sequence
[ReceiveStreamDecoder
],None
]) – Stream MIMO coding configuration during signal reception.encoding (
Union
[EncoderManager
,Sequence
[Encoder
],None
]) – Bit coding configuration.waveform (
Optional
[TypeVar
(CWT
, bound= CommunicationWaveform)]) – The waveform to be transmitted by this modem.frame_generator (
FrameGenerator
|None
) – Frame generator used to pack and unpack communication frames. If not specified, a stub generator will be assumed.seed (
int
|None
) – Seed used to initialize the pseudo-random number generator.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- property receive_signal_coding: ReceiveSignalCoding¶
Stream MIMO coding configuration during signal reception.
- property receive_symbol_coding: ReceiveSymbolCoding¶
Complex communication symbol coding configuration during reception.