SionnaRT Channel¶
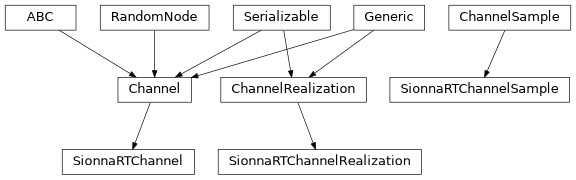
The SionnaRTChannel
is an adapter for Sionna Ray Tracing module.
It is deterministic, defined by the given sionna.rt.Scene. Meaning, given same devices and positions, different channel samples (SionnaRTChannelSample
) and realizations (SionnaRTChannelRealization
) would not introduce any random changes and would produce equal state and propagation results.
This channel model requires sionna.rt.Scene to operate. It should be loaded with sionna.rt.load_scene and provided to the SionnaRTChannel
__init__ method.
Current assumptions in the adapter implementation are:
All the transmitting and receiving antennas utilize isometric pattern (“iso”) and a single vertical polarization (“V”).
The antenna arrays are synthetic.
The delays are not normalized.
If not a single ray hit was cought, then a zeroed signal or state are returned.
It should be noted that sionna.rt.Scene is a singleton class. So when a new scene is loaded, a conflict with the previous existing instance will occure. Thus usage of several scenes at once must be avoided.
classDiagram direction LR class SionnaRTChannel { _realize() : SionnaRTChannelRealization } class SionnaRTChannelRealization { _sample() : SionnaRTChannelSample } class SionnaRTChannelSample { propagate(Signal) : Signal } SionnaRTChannel --o SionnaRTChannelRealization : realize() SionnaRTChannelRealization --o SionnaRTChannelSample : sample() click SionnaRTChannel href "#hermespy.channel.sionna_rt_channel.SionnaRTChannel" click SionnaRTChannelRealization href "#hermespy.channel.sionna_rt_channel.SionnaRTChannelRealization" click SionnaRTChannelSample href "#hermespy.channel.sionna_rt_channel.SionnaRTChannelSample"
The following minimal example outlines how to configure the channel model
within the context of a Simulation
:
1# Initialize two devices to be linked by a channel
2simulation = Simulation()
3alpha_device = simulation.new_device(
4 carrier_frequency=24e9, pose=Transformation.From_Translation(np.array([8.5, 21., 27.])))
5beta_device = simulation.new_device(
6 carrier_frequency=24e9, pose=Transformation.From_Translation(np.array([45., 90., 1.5])))
7
8# Load the desired scene
9scene = rt.load_scene(rt.scene.munich)
10
11# Create a channel between the two devices
12channel = SionnaRTChannel(scene=scene, gain=1., seed=42)
13simulation.set_channel(alpha_device, beta_device, channel)
14
15# Configure communication link between the two devices
16link = SimplexLink()
17alpha_device.transmitters.add(link)
18beta_device.receivers.add(link)
19
20# Specify the waveform and postprocessing to be used by the link
21link.waveform = RRCWaveform(
22 symbol_rate=1e8, oversampling_factor=2, num_data_symbols=1000,
23 num_preamble_symbols=10, pilot_rate=10)
24link.waveform.channel_estimation = SCLeastSquaresChannelEstimation()
25link.waveform.channel_equalization = SCZeroForcingChannelEqualization()
- class SionnaRTChannel(scene, gain=1.0, seed=None)[source]¶
Bases:
Channel
[SionnaRTChannelRealization
,SionnaRTChannelSample
]Sionna ray-tracing channel.
Refer to SionnaRT Channel for further information.
- Parameters:
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- _realize()[source]¶
Generate a new channel realzation.
Abstract subroutine of
realize
. EachChannel
is required to implement their own_realize()
method.Returns: A new channel realization.
- Return type:
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- class SionnaRTChannelRealization(scene, scene_file, sample_hooks=None, gain=1.0)[source]¶
Bases:
ChannelRealization
[SionnaRTChannelSample
]Realization of a Sionna RT channel.
Generated by the
_realize()
routine ofSionnaRTChannels
.- Parameters:
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- _sample(state)[source]¶
Sample the channel realization at a given point in time and space.
Abstract subroutine of
sample
.- Parameters:
state (
LinkState
) – State of the channel at the time of sampling.- Return type:
Returns: The channel sample at the given point in time.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- class SionnaRTChannelSample(paths, gain, state)[source]¶
Bases:
ChannelSample
Sample of a Sionna RT channel realization.
Generated by sampling a
SionnaRTChannelRealization
.- Parameters:
- state(num_samples, max_num_taps, interpolation_mode=InterpolationMode.NEAREST)[source]¶
Generate the discrete channel state information from this channel realization.
Denoted by
\[\mathbf{H}^{(m, \tau)} \in \mathbb{C}^{N_{\mathrm{Rx}} \times N_{\mathrm{Tx}}}\]within the respective equations.
- Parameters:
num_samples (
int
) – Number of discrete time-domain samples of the chanel state information.max_num_taps (
int
) – Maximum number of delay taps considered per discrete time-domain sample.interpolation_mode (
InterpolationMode
) – Interpolation behaviour of the channel realization’s delay components with respect to the proagated signal’s sampling rate. If not specified, an integer rounding to the nearest sampling instance will be assumed.
- Return type:
Returns: The channel state information representing this channel realization.
- property expected_energy_scale: float¶
Expected linear scaling of a propagated signal’s energy at each receiving antenna.
Required to compute the expected energy of a signal after propagation, and therfore signal-to-noise ratios (SNRs) and signal-to-interference-plus-noise ratios (SINRs).
TODO Current implementation is technically incorrect.