Exponential¶
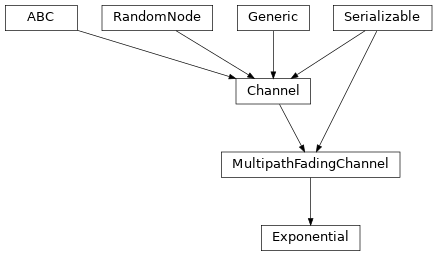
Exponentially decaying channel model.
The following minimal example outlines how to configure the channel model
within the context of a Simulation
:
1# Initialize two devices to be linked by a channel
2simulation = Simulation()
3alpha_device = simulation.new_device(carrier_frequency=1e8)
4beta_device = simulation.new_device(carrier_frequency=1e8)
5
6# Create a channel between the two devices
7channel = Exponential(1e-8, 1e-7)
8simulation.set_channel(alpha_device, beta_device, channel)
9
10# Configure communication link between the two devices
11link = SimplexLink()
12alpha_device.transmitters.add(link)
13beta_device.receivers.add(link)
14
15# Specify the waveform and postprocessing to be used by the link
16link.waveform = RRCWaveform(
17 symbol_rate=1e8, oversampling_factor=2, num_data_symbols=1000,
18 num_preamble_symbols=10, pilot_rate=10)
19link.waveform.channel_estimation = SCLeastSquaresChannelEstimation()
20link.waveform.channel_equalization = SCZeroForcingChannelEqualization()
21
22# Configure a simulation to evaluate the link's BER and sweep over the receive SNR
23simulation.add_evaluator(BitErrorEvaluator(link, link))
24simulation.new_dimension('noise_level', dB(0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20), beta_device)
25
26# Run simulation and plot resulting SNR curve
- class Exponential(tap_interval, rms_delay, gain=1.0, **kwargs)[source]¶
Bases:
MultipathFadingChannel
Exponential multipath fading channel model.
- Parameters:
tap_interval (float) – Tap interval in seconds.
rms_delay (float) – Root-Mean-Squared delay in seconds.
gain (float, optional) – Linear power gain factor a signal experiences when being propagated over this realization. \(1.0\) by default.
**kwargs (Any) – MultipathFadingChannel initialization parameters.
- Raises:
ValueError – On invalid arguments.