Audio Device¶
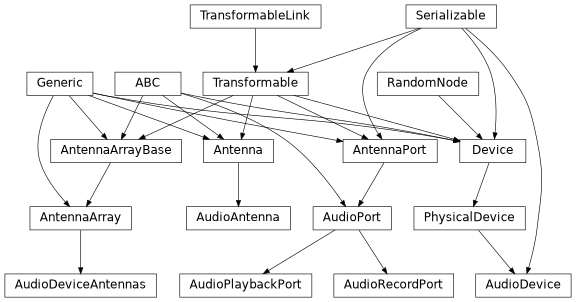
Hermes hardware bindings to audio devices offer the option to benchmark complex-valued communication waveforms over affordable consumer-grade audio hardware. The effective available bandwidth is limited to half of the audio devices sampling rate, which is typically either \(44.1~\\mathrm{kHz}\) or \(48~\\mathrm{kHz}\).
- class AudioDevice(playback_device, record_device, playback_channels=None, record_channels=None, sampling_rate=48000.0, **kwargs)[source]¶
Bases:
PhysicalDevice
[PhysicalDeviceState
],Serializable
HermesPy binding to an arbitrary audio device. Let’s rock!
- Parameters:
playback_device (
int
) – Device over which audio streams are to be transmitted.record_device (
int
) – Device over which audio streams are to be received.playback_channels (
Optional
[Sequence
[int
]]) – List of audio channels for signal transmission. By default, the first channel is selected.record_channels (
Optional
[Sequence
[int
]]) – List of audio channels for signal reception. By default, the first channel is selected.sampling_rate (
float
) – Configured sampling rate. 48 kHz by default.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- state()[source]¶
Query the immutable physical state of the device.
Returns: The physical device state.
- Return type:
- property antennas: AudioDeviceAntennas¶
Model of the device’s antenna array.
- property carrier_frequency: float¶
Central frequency of the device’s emissions in the RF-band.
Denoted by \(f_c\) with unit \(\left[ f_c \right] = \mathrm{Hz} = \tfrac{1}{\mathrm{s}}\) in the literature.
- Raises:
ValueError – On negative carrier frequencies.
- property playback_channels: Sequence[int]¶
Audio channels for signal transmission.
Returns: Sequence of audio channel indices.
- Raises:
ValueError – On arguments not representing vectors.
- property playback_device: int¶
Device over which audio streams are to be transmitted.
Returns: Device identifier.
- Raises:
ValueError – For negative identifiers.
- property record_channels: Sequence[int]¶
Audio channels for signal reception.
Returns: Sequence of audio channel indices.
- Raises:
ValueError – On arguments not representing vectors.
- property record_device: int¶
Device over which audio streams are to be received.
Returns: Device identifier.
- Raises:
ValueError – For negative identifiers.
- property sampling_rate: float¶
Sampling rate at which the device’s analog-to-digital converters operate in Hz.
- Raises:
ValueError – If the sampling rate is not greater than zero.
- class AudioAntenna(mode=AntennaMode.DUPLEX, pose=None)[source]¶
Bases:
Antenna
Antenna model for audio devices.
- Parameters:
mode (
AntennaMode
) – Antenna’s mode of operation. By default, a full duplex antenna is assumed.pose (
Transformation
|None
) – The antenna’s position and orientation with respect to its array.
- copy()[source]¶
Create a deep copy of the antenna.
- Return type:
- Returns:
A deep copy of the antenna.
- local_characteristics(azimuth, elevation)[source]¶
Generate a single sample of the antenna’s characteristics.
The polarization is characterized by the angle-dependant field vector
\[\begin{split}\mathbf{F}(\phi, \theta) = \begin{pmatrix} F_{\mathrm{H}}(\phi, \theta) \\ F_{\mathrm{V}}(\phi, \theta) \\ \end{pmatrix}\end{split}\]denoting the horizontal and vertical field components. The directional antenna gain can be computed from the polarization vector magnitude
\[\begin{split}A(\phi, \theta) &= \lVert \mathbf{F}(\phi, \theta) \rVert \\ &= \sqrt{ F_{\mathrm{H}}(\phi, \theta)^2 + F_{\mathrm{V}}(\phi, \theta)^2 }\end{split}\]- Parameters:
azimuth (
float
) – Considered horizontal wave angle in radians \(\phi\).elevation – Considered vertical wave angle in radians \(\theta\).
- Return type:
- Returns:
Two dimensional numpy array denoting the horizontal and vertical ploarization components of the antenna response vector.
- class AudioPort(antennas=None, pose=None, array=None)[source]¶
Bases:
ABC
,AntennaPort
[AudioAntenna
,AudioDeviceAntennas
]Antenna port model for audio devices.
- Parameters:
antennas (
Sequence
[TypeVar
(AT
, bound=Antenna
)] |None
) – Sequence of antennas to be connected to this port. If not specified, no antennas are connected by default.pose (
Transformation
|None
) – The antenna port’s position and orientation with respect to its array.array (
Optional
[TypeVar
(AAT
, bound= AntennaArray)]) – Antenna array this port belongs to.
- class AudioPlaybackPort(array, channel)[source]¶
Bases:
AudioPort
Antenna transmit port model for audio devices.
Represents a single transmitting audio channel of a soundcard.
- Parameters:
array (
AudioDeviceAntennas
) – Antenna array to which the port belongs.channel (
int
) – Recording audio channel index.
- class AudioRecordPort(array, channel)[source]¶
Bases:
AudioPort
Antenna receive port model for audio devices.
Represents a single receiving audio channel of a soundcard.
- Parameters:
array (
AudioDeviceAntennas
) – Antenna array to which the port belongs.channel (
int
) – Recording audio channel index.
- class AudioDeviceAntennas(device)[source]¶
Bases:
AntennaArray
[AudioPort
,AudioAntenna
]Antenna array information for audio devices.
- Parameters:
pose – The antenna array’s position and orientation with respect to its device. If not specified, the same orientation and position as the device is assumed.
- property receive_ports: Sequence[AudioRecordPort]¶
Sequence of all receiving ports within this array.
- property transmit_ports: Sequence[AudioPlaybackPort]¶
Sequence of all transmitting ports within this array.