Serialization Factory¶
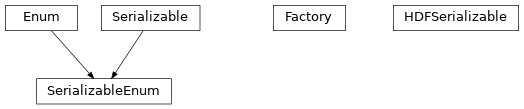
This module implements the main interface for loading / dumping HermesPy configurations from / to YAML files.
Every mutable object that is expected to have its state represented as a text-section within configuration files
must inherit from the Serializable
base class.
All Serializable
classes within the hermespy namespace are detected automatically by the Factory
managing the serialization process.
As a result, dumping any Serializable
object state to a .yml text file is as easy as
factory = Factory()
factory.to_file("dump.yml", serializable)
and can be loaded again just as easily via
factory = Factory()
serializable = factory.from_file("dump.yml")
from any context.
- class Serializable[source]¶
Bases:
object
Base class for serializable classes.
Only classes inheriting from Serializable will be serialized by the factory.
- class SerializableEnum(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)[source]¶
Bases:
Serializable
,Enum
Base class for serializable enumerations.
- class HDFSerializable[source]¶
Bases:
object
Base class for object serializable to the HDF5 format.
Structures are serialized to HDF5 files by the
to_HDF
routine and de-serialized by thefrom_HDF
method, respectively.
- class Factory[source]¶
Bases:
object
Helper class to load HermesPy simulation scenarios from YAML configuration files.
- from_file(file)[source]¶
Load a configuration from a single YAML file.
Returns: Serialized objects within path.
- from_folder(path, recurse=True, follow_links=False)[source]¶
Load a configuration from a folder.
- Parameters:
- Return type:
Returns: Serializable objects recalled from path.
- Raises:
ValueError – If path is not a directory.
- from_path(paths)[source]¶
Load a configuration from an arbitrary file system path.
- Parameters:
paths (Union[str, Set[str]]) – Paths to a file or a folder featuring .yml config files.
- Return type:
Returns: Serializable objects recalled from paths.
- Raises:
ValueError – If the provided path does not exist on the filesystem.
- from_str(config)[source]¶
Load a configuration from a string object.
Returns: List of objects or object from config.
- to_str(*args)[source]¶
Dump a configuration to a folder.
- Parameters:
*args (Any) – Configuration objects to be dumped.
- Returns:
String containing full YAML configuration.
- Return type:
- Raises:
RepresenterError – If objects in
*args
are unregistered classes.
- to_stream(stream, *args)[source]¶
Dump a configuration to an arbitrary text stream.
- Parameters:
stream (TextIOBase) – Text stream to the configuration.
*args (Any) – Configuration objects to be dumped.
- Raises:
RepresenterError – If objects in
*args
are unregistered classes.- Return type:
- property clean: bool¶
Use clean YAML standard.
Disabling the clean flag will deactivate additional text processing to the YAML configuration files done by Hermes, such as dB conversion or linear number spaces.
Returns: Clean flag.
-
extensions:
Set
[str
] = {'.cfg', '.yaml', '.yml'}¶ List of recognized filename extensions for serialization files.
- property registered_classes: ValuesView[Type[Serializable]]¶
Classes registered for serialization within the factory.
- property tag_registry: Mapping[str, Type[Serializable]]¶
Read registered YAML tags.
- class SerializableType¶
Type of Serializable Class.
alias of TypeVar(‘SerializableType’, bound=
Serializable
)
- class SET¶
Type of serializable enumeration.
alias of TypeVar(‘SET’, bound=
SerializableEnum
)