Waveform¶
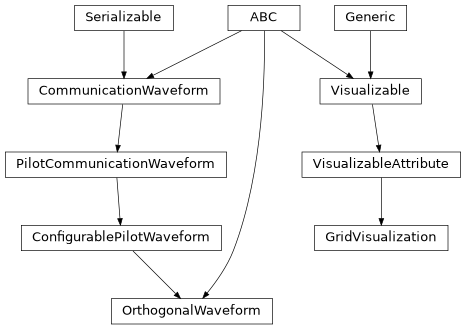
- class OrthogonalWaveform(num_subcarriers, grid_resources, grid_structure, pilot_section=None, pilot_sequence=None, repeat_pilot_sequence=True, **kwargs)[source]¶
Bases:
ConfigurablePilotWaveform
,ABC
Base class for wavforms with orthogonal subcarrier modulation.
- Parameters:
num_subcarriers (
int
) – Number of available orthogonal subcarriers per symbol.grid_resources (
Sequence
[GridResource
]) – Grid resources available for modulation.grid_structure (
Sequence
[GridSection
]) – Grid structure of the time-domain.pilot_section (
PilotSection
|None
) – Pilot section transmitted at the beginning of each frame.pilot_sequence (
PilotSymbolSequence
|None
) – Sequence of pilot / reference symbols.repeat_pilot_sequence (
bool
) – Repeat the pilot sequence if it is shorter than the frame.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- map(data_bits)[source]¶
Map a stream of bits to data symbols.
- Parameters:
data_bits (
ndarray
) – Vector containing a sequence of L hard data bits to be mapped onto data symbols.- Return type:
Returns: Mapped data symbols.
- modulate(symbols)[source]¶
Modulate a stream of data symbols to a base-band signal containing a single data frame.
- Parameters:
data_symbols – Singular stream of data symbols to be modulated by this waveform.
- Return type:
Returns: Samples of the modulated base-band signal.
- pick(placed_symbols)[source]¶
Pick the mapped symbols from the communicaton frame.
Additionally removes interleaved pilot symbols.
- Parameters:
placed_symbols (
StatedSymbols
) – The placed symbols.- Return type:
Returns: The symbols with the mapped symbols picked from the frame.
- place(symbols)[source]¶
Place the mapped symbols within the communicaton frame.
Additionally interleaves pilot symbols.
Returns: The symbols with the mapped symbols placed within the frame.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- property bit_energy: float¶
Returns the theoretical average (discrete-time) bit energy of the modulated baseband_signal.
Energy of baseband_signal \(x[k]\) is defined as \(\sum{|x[k]}^2\) Only data bits are considered, i.e., reference, guard intervals are ignored.
- property grid_resources: Sequence[GridResource]¶
Available resources within the time-subcarrier grid.
- property grid_structure: Sequence[GridSection]¶
Structure of the time-subcarrier grid.
- property mapping: PskQamMapping¶
Constellation mapping used to translate bit sequences into complex symbols.
- property modulation_order: int¶
Access the modulation order.
- Returns:
The modulation order.
- Return type:
- property num_data_symbols: int¶
Number of bit-mapped symbols contained within each communication frame.
- property num_subcarriers: int¶
Number of available orthogonal subcarriers.
- Raises:
ValueError – If smaller than one.
- property pilot_section: PilotSection | None¶
Static pilot section transmitted at the beginning of each OFDM frame.
Required for time-domain synchronization and equalization of carrier frequency offsets.
- Returns:
FrameSection of the pilot symbols, None if no pilot is configured.
- property pilot_signal: Signal¶
Model of the pilot sequence within this communication waveform.
Returns: The pilot sequence.
- property plot_grid: GridVisualization¶
Visualize the resource grid.
- property power: float¶
Returns the theoretical average symbol (unitless) power,
Power of baseband_signal \(x[k]\) is defined as \(\sum_{k=1}^N{|x[k]|}^2 / N\) Power is the average power of the data part of the transmitted frame, i.e., bit energy x raw bit rate
- property symbol_energy: float¶
The theoretical average symbol (discrete-time) energy of the modulated baseband_signal.
Energy of baseband_signal \(x[k]\) is defined as \(\sum{|x[k]}^2\) Only data bits are considered, i.e., reference, guard intervals are ignored.
- Returns:
The average symbol energy in UNIT.
- class OWT¶
Type variable for orthogonal waveform types.
alias of TypeVar(‘OWT’, bound=
OrthogonalWaveform
)
- class GridVisualization(wave)[source]¶
Bases:
VisualizableAttribute
[ImageVisualization
]Plot the grid structure of an orthogonal waveform.
- Parameters:
wave (
OrthogonalWaveform
) – Waveform this plot is associated with.