Device Model¶
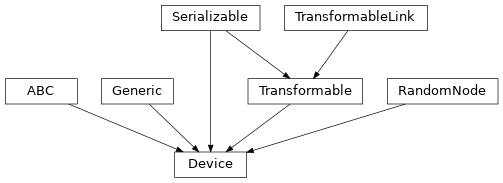
- class Device(transmit_dsp=None, receive_dsp=None, transmit_encoding=None, receive_decoding=None, power=1.0, pose=None, seed=None)[source]¶
Bases:
ABC
,Generic
[DST
],Transformable
,RandomNode
,Serializable
Physical device representation within HermesPy.
It acts as the basis for all transmissions and receptions of sampled electromagnetic signals.
- Parameters:
transmit_dsp (
Transmitter
|Sequence
[Transmitter
] |None
) – Digital transmit signal processing algorithms operating on this device.receive_dsp (
Receiver
|Sequence
[Receiver
] |None
) – Digital receive signal processing algorithms operating on this device.transmit_encdoding – Digital coding applied to transmitted samples before digital-to-analog conversion.
receive_decoding (
ReceiveSignalCoding
|Sequence
[ReceiveStreamDecoder
] |ReceiveStreamDecoder
|None
) – Digital coding applied to received samples after analog-to-digital conversion.power (
float
) – Average power of the transmitted signals in Watts. 1.0 Watt by default.pose (
Transformation
|None
) – Pose of the device with respect to its scenario coordinate system origin.seed (
int
|None
) – Random seed used to initialize the pseudo-random number generator.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
TypeVar
(DeviceType
, bound= Device)- Returns:
The deserialized object.
- add_dsp(dsp)[source]¶
Add a DSP algorithm to this device.
- Parameters:
dsp (
Transmitter
|Receiver
) – DSP algorithm to be added to the device. If the DSP algorithm is a transmitter, it will be added to the transmit layer. If the DSP algorithm is a receiver, it will be added to the receive layer. If the DSP is both, it will be added to both layers.- Return type:
- generate_output(operator_transmissions, state=None, resample=True)[source]¶
Generate the device’s output.
- Parameters:
operator_transmissions (
Sequence
[Transmission
]) – List of operator transmissions from which to generate the output.state (
Optional
[TypeVar
(DST
, bound=DeviceState
)]) – Device state to be used for the output generation. If not provided, query the current device stateresample (
bool
) – Resample the output signal to the device’s sampling rate. Enabled by default.
- Return type:
Returns: The device’s output.
- Raises:
RuntimeError – If the transmit coding is incompatible with the number of transmit antenna ports.
- process_input(impinging_signals, state=None)[source]¶
Process input signals impinging onto this device.
- Parameters:
impinging_signals (
DeviceInput
|Signal
|Sequence
[Signal
]) – The samples to be processed by the device.state (
Optional
[TypeVar
(DST
, bound=DeviceState
)]) – Device state to be used for the processing. If not provided, query the current device state.
- Return type:
Returns: The processed device input information.
- Raises:
ValueError – If the number of signal streams does not match the device configuration expectations.
- receive(impinging_signals, state=None, notify=True)[source]¶
Receive over this device.
Internally calls
Device.process_input()
andDevice.receive_operators()
.- Parameters:
impinging_signals (
DeviceInput
|Signal
|Sequence
[Signal
]) – The samples to be processed by the device.state (
Optional
[TypeVar
(DST
, bound=DeviceState
)]) – Device state to be used for the processing. If not provided, query the current device state by callingstate
.notify (
bool
) – Notify all registered callbacks within the involved DSP algorithms. Enabled by default.
- Return type:
Returns: The received device information.
- receive_operators(operator_inputs, state=None, notify=True)[source]¶
Receive over the registered operators.
- Parameters:
operator_inputs (
ProcessedDeviceInput
|Sequence
[Signal
]) – The signal samples to be processed by the device’s operators. If aProcessedDeviceInput
is provided, the operator inputs will be extracted from it. Otherwise, the operator inputs are assumed to be provided directly.state (
Optional
[TypeVar
(DST
, bound=DeviceState
)]) – Assumed state of the device during reception. If not provided, the current device state will be queried by callingstate()
.notfiy – Notify all registered callbacks within the involved DSP algorithms. Enabled by default.
- Return type:
Returns: List of information generated by receiving over the device’s operators.
- Raises:
ValueError – If the number of operator inputs does not match the number of receive operators.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- abstract state()[source]¶
Query the immutable physical state of the device.
Returns: The physical device state.
- Return type:
TypeVar
(DST
, bound=DeviceState
)
- transmit(state=None, notify=True)[source]¶
Transmit over this device.
- Parameters:
state (
Optional
[TypeVar
(DST
, bound=DeviceState
)]) – Device state to be used for the transmission. If not provided, query the current device state by callingstate
.notify (
bool
) – Notify the transmitter’s callbacks about the generated transmission. Enabled by default.
- Return type:
Returns: Information transmitted by this device.
- transmit_operators(state=None, notify=True)[source]¶
Generate transmitted information for all registered operators.
Calls
Transmitter.transmit()
for each transmit operator.- Parameters:
state (
Optional
[TypeVar
(DST
, bound=DeviceState
)]) – Device state to be used for the transmission. If not provided, query the current device state by callingstate()
.notify (
bool
) – Notify the transmitter’s callbacks about the generated transmission. Enabled by default.
- Return type:
Returns: List of operator transmisisons.
- abstract property antennas: AntennaArray¶
Model of the device’s antenna array.
- abstract property carrier_frequency: float¶
Central frequency of the device’s emissions in the RF-band.
Denoted by \(f_c\) with unit \(\left[ f_c \right] = \mathrm{Hz} = \tfrac{1}{\mathrm{s}}\) in the literature.
- Raises:
ValueError – On negative carrier frequencies.
- property input_callbacks: Hookable[ProcessedDeviceInput]¶
Callbacks notified about processed inputs.
- property num_digital_receive_ports: int¶
Number of available digital signal stream ports for reception.
If no receive coding was configured, this value is equal to the number of receive antenna ports.
- property num_digital_transmit_ports: int¶
Number of available digital signal stream ports for transmission.
If no transmit coding was configured, this value is equal to the number of transmit antenna ports.
- property num_receive_antenna_ports: int¶
Number of available receive antenna ports.
Short hand to
antennas'
num_receive_ports
.
- property num_receive_antennas: int¶
Number of available receive antennas.
Shorthand to
antennas'
num_receive_antennas
.
- property num_transmit_antenna_ports: int¶
Number of available transmit antenna ports.
Shorthand to
antennas'
num_transmit_ports
.
- property num_transmit_antennas: int¶
Number of available transmit antennas.
Shorthand to
antennas'
num_transmit_antennas
.
- property output_callbacks: Hookable[DeviceOutput]¶
Callbacks notified about generated outputs.
- property power: float¶
Average power of the transmitted signal signal in \(\mathrm{V}^2\).
- Raises:
ValueError – If value is smaller than zero.
- property receive_coding: ReceiveSignalCoding¶
Digital coding applied to received samples after analog-to-digital conversion.
- receivers: ReceiverSlot¶
Receivers capturing signals from this device
- abstract property sampling_rate: float¶
Sampling rate at which the device’s analog-to-digital converters operate in Hz.
- Raises:
ValueError – If the sampling rate is not greater than zero.
- property transmit_coding: TransmitSignalCoding¶
Digital coding applied to transmitted samples before digital-to-analog conversion.
- transmitters: TransmitterSlot¶
Transmitters broadcasting signals over this device.
- property wavelength: float¶
Central wavelength of the device’s emmissions in the RF-band in m.
Denoted by \(\lambda\) with unit \(\left[ \lambda \right] = \mathrm{m}\) in the literature. Related to the carrier frequency by the speed of light \(c\) so that \(\lambda = \frac{c}{f_c}\).
- Raises:
ValueError – On wavelengths smaller or equal to zero.