5G TDL¶
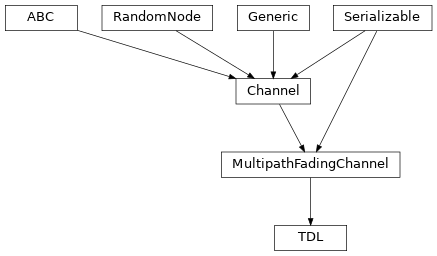
Implementation of the 3GPP standard parameterizations as stated in ETSI TR 38.900 [1]. Five scenario types A-E are defined, differing in the number of considered paths and the path’s respective delay and power.
The following minimal example outlines how to configure the channel model
within the context of a Simulation
:
1# Initialize two devices to be linked by a channel
2simulation = Simulation()
3alpha_device = simulation.new_device(carrier_frequency=1e8)
4beta_device = simulation.new_device(carrier_frequency=1e8)
5
6# Create a channel between the two devices
7channel = TDL(model_type=TDLType.B, rms_delay=1e-9)
8simulation.set_channel(alpha_device, beta_device, channel)
9
10# Configure communication link between the two devices
11link = SimplexLink()
12alpha_device.transmitters.add(link)
13beta_device.receivers.add(link)
14
15# Specify the waveform and postprocessing to be used by the link
16link.waveform = RRCWaveform(
17 symbol_rate=1e8, oversampling_factor=2, num_data_symbols=1000,
18 num_preamble_symbols=10, pilot_rate=10
19)
20link.waveform.channel_estimation = SCLeastSquaresChannelEstimation()
21link.waveform.channel_equalization = SCZeroForcingChannelEqualization()
22
23# Configure a simulation to evaluate the link's BER and sweep over the receive SNR
24simulation.add_evaluator(BitErrorEvaluator(link, link))
25simulation.new_dimension('noise_level', dB(0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20), beta_device)
26
27# Run simulation and plot resulting SNR curve
- class TDL(model_type=TDLType.A, rms_delay=0.0, correlation_distance=inf, num_sinusoids=20, los_angle=None, doppler_frequency=0.0, los_doppler_frequency=None, antenna_correlation=None, gain=1.0, seed=None)[source]¶
Bases:
MultipathFadingChannel
5G TDL Multipath Fading Channel models.
- Parameters:
model_type (
TDLType
) – The model type. If not specified, the default model type A is assumed.rms_delay (
float
) – Root-Mean-Squared delay in seconds. Initializes therms_delay
attribute.correlation_distance (
float
) – Distance at which channel samples are considered to be uncorrelated. \(\infty\) by default, i.e. the channel is considered to be fully correlated in space.num_sinusoids (
int
) – Number of sinusoids used to sample the statistical distribution. Denoted by \(N\) within the respective equations.los_angle (
float
|None
) – Angle phase of the line of sight component within the statistical distribution.doppler_frequency (
float
) – Doppler frequency shift of the statistical distribution. Denoted by \(\omega_{\ell}\) within the respective equations.antenna_correlation (
AntennaCorrelation
|None
) – Antenna correlation model. By default, the channel assumes ideal correlation, i.e. no cross correlations.gain (
float
) – Linear power gain factor a signal experiences when being propagated over this realization. \(1.0\) by default.seed (
int
|None
) – Seed used to initialize the pseudo-random number generator.
- Raises:
ValueError – If rms_delay is smaller than zero.
ValueError – If los_angle is specified in combination with model_type D or E.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- class TDLType(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)[source]¶
Bases:
SerializableEnum
Supported model types of the 5G TDL channel model
- A = 0¶
- B = 1¶
- C = 2¶
- D = 4¶
- E = 5¶