USRP Device¶
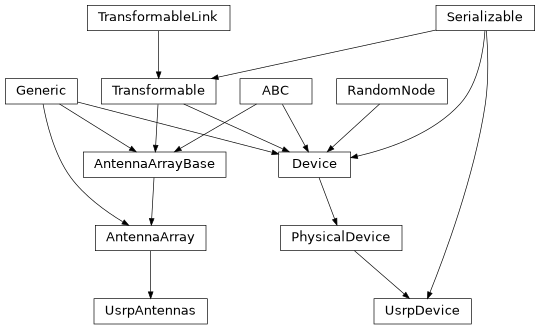
- class UsrpDevice(ip, port=5555, carrier_frequency=700000000.0, sampling_rate=None, tx_gain=0.0, rx_gain=0.0, scale_transmission=True, num_prepended_zeros=200, num_appended_zeros=200, selected_transmit_ports=None, selected_receive_ports=None, **kwargs)[source]¶
Bases:
PhysicalDevice
[PhysicalDeviceState
],Serializable
Bindung to a USRP device via the UHD library.
- Parameters:
ip (
str
) – The IP address of the USRP device.port (
int
) – The port of the USRP device.carrier_frequency (
float
) – Carrier frequency of the USRP device. \(700~\mathrm{MHz}\) by default.sampling_rate (
float
|None
) – Sampling rate of the USRP device. If not provided, the sampling rate is determined from the configured operators.tx_gain (
float
) – The transmission gain of the USRP device. Zero by default.rx_gain (
float
) – The reception gain of the USRP device. Zero by default.scale_transmission (
bool
) – If True, the transmission signal is scaled to the maximum floating point value of the USRP device. This ensures a proper digital to analog conversion.num_prepended_zeros (
int
) – The number of zeros prepended to the transmission signal. \(200\) by default.num_appended_zeros (
int
) – The number of zeros appended to the transmission signal. \(200\) by default.selected_transmit_ports (
Sequence
[int
] |None
) – Indices of the selected transmit antenna ports. If not specified, i.e.None
, only the first antenna port is selected.selected_receive_ports (
Sequence
[int
] |None
) – Indices of the selected receive antenna ports. If not specified, i.e.None
, only the first antenna port is selected.**kwargs – Additional arguments passed to the
PhysicalDevice
parent class.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- state()[source]¶
Query the immutable physical state of the device.
Returns: The physical device state.
- Return type:
- property antennas: UsrpAntennas¶
Antenna array model of the USRP device.
Allows for the further configuration of the antenna elements, however, the number of
transmit ports
andreceive ports
is fixed.
- property carrier_frequency: float¶
Central frequency of the device’s emissions in the RF-band.
Denoted by \(f_c\) with unit \(\left[ f_c \right] = \mathrm{Hz} = \tfrac{1}{\mathrm{s}}\) in the literature.
- Raises:
ValueError – On negative carrier frequencies.
- property num_appended_zeros: int¶
Number of zero padding samples appended to the transmitted signal.
Returns: Number of appended samples.
- Raises:
ValueError – For negative values.
- property num_prepeneded_zeros: int¶
Number of zero padding samples prepended to the transmitted signal.
Returns: Number of prepended samples.
- Raises:
ValueError – For negative values.
- property sampling_rate: float¶
Sampling rate at which the device’s analog-to-digital converters operate in Hz.
- Raises:
ValueError – If the sampling rate is not greater than zero.
- class UsrpAntennas(device, pose=None)[source]¶
Bases:
AntennaArray
[AntennaPort
,Antenna
]Antenna and port configuration of a USRP device.
UsrpAntennas
’ port configuration is linked to the attached :class`UsrpDevice` and cannot be changed manually.- Parameters:
device (
UsrpDevice
) – USRP device this antenna array models.pose (
Transformation
|None
) – Pose of the antenna array with respect to the device.
- property device: UsrpDevice¶
USRP device the antenna array is attached to.
- property ports: Sequence[AntennaPort]¶
Sequence of all antenna ports within this array.
- property receive_ports: Sequence[AntennaPort]¶
Sequence of all receiving ports within this array.
- property transmit_ports: Sequence[AntennaPort]¶
Sequence of all transmitting ports within this array.