Precoding¶
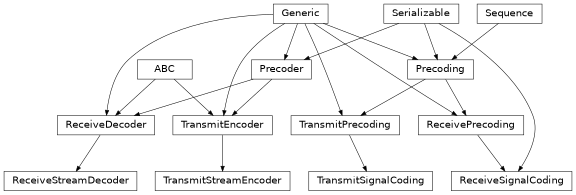
Precodings are HermesPy’s way of representing a sequence of operations on parallel complex-valued data streams.
- class Precoder[source]¶
Bases:
Generic
[PrecodingType
],Serializable
Base class for signal processing algorithms operating on parallel complex data streams.
- property precoding: PrecodingType | None¶
Access the precoding configuration this precoder is attached to.
- Returns:
Handle to the precoding. None if the precoder is considered floating.
- Raises:
RuntimeError – If this precoder is currently floating.
- class TransmitEncoder[source]¶
Bases:
ABC
,Precoder
[TransmitPrecodingType
],Generic
[TransmitPrecodingType
]Base class of precoding steps within transmit precoding configurations.
- class ReceiveDecoder[source]¶
Bases:
ABC
,Precoder
[ReceivePrecodingType
],Generic
[ReceivePrecodingType
]Base class of precoding steps within receive precoding configurations.
- class Precoding[source]¶
Bases:
Sequence
[PrecoderType
],Generic
[PrecoderType
],Serializable
Base class of precoding configurations.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- pop_precoder(index)[source]¶
Remove a precoder from the processing chain.
- Parameters:
index (int) – Index of the precoder to be removed.
- Return type:
TypeVar
(PrecoderType
, bound= Precoder)
Returns: Handle to the removed precoder.
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- class TransmitPrecoding[source]¶
Bases:
Precoding
[TransmitEncoderType
],Generic
[TransmitEncoderType
]Base class for transmit encoding configurations.
- class ReceivePrecoding[source]¶
Bases:
Precoding
[ReceiveDecoderType
],Generic
[ReceiveDecoderType
]Base class for receive decoding configurations.
- class TransmitStreamEncoder[source]¶
Bases:
TransmitEncoder
[TransmitSignalCoding
]Base class for multi-stream MIMO coding steps during signal transmission.
- abstract encode_streams(streams, num_output_streams, device)[source]¶
Encode a signal MIMO stream during transmission.
- Parameters:
streams (Signal) – The signal stream to be encoded.
num_output_streams (int) – Number of desired output streams.
device (TransmitState) – Physical state of the device.
- Return type:
Returns: The encoded signal stream.
- class ReceiveStreamDecoder[source]¶
Bases:
ReceiveDecoder
[ReceiveSignalCoding
]Base class for multi-stream MIMO coding steps during signal reception.
- abstract decode_streams(streams, num_output_streams, device)[source]¶
Encode a signal MIMO stream during signal recepeption.
- Parameters:
streams (Signal) – The signal stream to be decoded.
num_output_streams (int) – Number of desired output streams.
device (ReceiveState) – Physical state of the device.
- Return type:
Returns: The decoded signal stream.
- class TransmitSignalCoding[source]¶
Bases:
TransmitPrecoding
[TransmitStreamEncoder
]Stream MIMO coding configuration during signal transmission.
- encode_streams(signal, device)[source]¶
Encode a signal MIMO stream during transmission.
This operation may modify the number of streams.
- Parameters:
streams (Signal) – The signal stream to be encoded.
device (TransmitState) – Physical state of the device.
- Return type:
Returns: The encoded signal stream.
- Raises:
ValueError – If the number of input streams does not match the configuration.
- class ReceiveSignalCoding[source]¶
Bases:
ReceivePrecoding
[ReceiveStreamDecoder
],Serializable
Stream MIMO coding configuration during signal transmission.
- decode_streams(signal, device)[source]¶
Decode a signal MIMO stream during reception.
This operation may modify the number of streams.
- Parameters:
streams (Signal) – The signal stream to be decoded.
device (ReceiveState) – Physical state of the device.
- Return type:
Returns: The decode signal stream.
- Raises:
ValueError – If the number of input streams does not match the configuration.
- class TransmitEncoderType¶
Type of transmit encoder.
alias of TypeVar(‘TransmitEncoderType’, bound=
TransmitEncoder
)
- class ReceiveDecoderType¶
Type of receive decoder.
alias of TypeVar(‘ReceiveDecoderType’, bound=
ReceiveDecoder
)
- class TransmitPrecodingType¶
Type of transmit precoding.
alias of TypeVar(‘TransmitPrecodingType’, bound=
TransmitPrecoding
)
- class ReceivePrecodingType¶
Type of receive precoding.
alias of TypeVar(‘ReceivePrecodingType’, bound=
ReceivePrecoding
)