Multipath Fading Channel¶
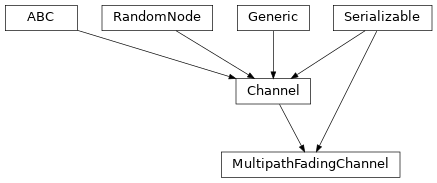
Allows for the direct configuration of the Multipath Fading Channel’s parameters
directly.
The following minimal example outlines how to configure the channel model
within the context of a Simulation
:
1# Initialize two devices to be linked by a channel
2simulation = Simulation()
3alpha_device = simulation.new_device(carrier_frequency=1e8)
4beta_device = simulation.new_device(carrier_frequency=1e8)
5
6# Create a channel between the two devices
7delays = 1e-9 * np.array([0, 0.3819, 0.4025, 0.5868, 0.4610, 0.5375, 0.6708, 0.5750, 0.7618, 1.5375, 1.8978, 2.2242, 2.1717, 2.4942, 2.5119, 3.0582, 4.0810, 4.4579, 4.5695, 4.7966, 5.0066, 5.3043, 9.6586])
8powers = 10 ** (np.array([-13.4, 0, -2.2, -4, -6, -8.2, -9.9, -10.5, -7.5, -15.9, -6.6, -16.7, -12.4, -15.2, -10.8, -11.3, -12.7, -16.2, -18.3, -18.9, -16.6, -19.9, -29.7]) / 10)
9rice_factors = np.zeros_like(delays)
10channel = MultipathFadingChannel(delays, powers, rice_factors)
11simulation.set_channel(alpha_device, beta_device, channel)
12
13# Configure communication link between the two devices
14link = SimplexLink()
15alpha_device.transmitters.add(link)
16beta_device.receivers.add(link)
17
18# Specify the waveform and postprocessing to be used by the link
19link.waveform = RRCWaveform(
20 symbol_rate=1e8, oversampling_factor=2, num_data_symbols=1000,
21 num_preamble_symbols=10, pilot_rate=10)
22link.waveform.channel_estimation = SCLeastSquaresChannelEstimation()
23link.waveform.channel_equalization = SCZeroForcingChannelEqualization()
24
25# Configure a simulation to evaluate the link's BER and sweep over the receive SNR
26simulation.add_evaluator(BitErrorEvaluator(link, link))
27simulation.new_dimension('noise_level', dB(0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20), beta_device)
28
29# Run simulation and plot resulting SNR curve
- class MultipathFadingChannel(delays, power_profile, rice_factors, correlation_distance=inf, num_sinusoids=20, los_angle=None, doppler_frequency=0.0, los_doppler_frequency=None, antenna_correlation=None, gain=1.0, seed=None)[source]¶
Bases:
Channel
[MultipathFadingRealization
,MultipathFadingSample
],Serializable
Base class for the implementation of stochastic multipath fading channels.
- Parameters:
delays (
Union
[ndarray
,List
[float
]]) – Delay in seconds of each individual multipath tap. Denoted by \(\tau_{\ell}\) within the respective equations.power_profile (
Union
[ndarray
,List
[float
]]) – Power loss factor of each individual multipath tap. Denoted by \(g_{\ell}\) within the respective equations.rice_factors (
Union
[ndarray
,List
[float
]]) – Rice factor balancing line of sight and multipath in each individual channel tap. Denoted by \(K_{\ell}\) within the respective equations.correlation_distance (
float
) – Distance at which channel samples are considered to be uncorrelated. \(\infty\) by default, i.e. the channel is considered to be fully correlated in space.num_sinusoids (
int
) – Number of sinusoids used to sample the statistical distribution. Denoted by \(N\) within the respective equations.los_angle (
float
|None
) – Angle phase of the line of sight component within the statistical distribution.doppler_frequency (
float
) – Doppler frequency shift of the statistical distribution. Denoted by \(\omega_{\ell}\) within the respective equations.antenna_correlation (
AntennaCorrelation
|None
) – Antenna correlation model. By default, the channel assumes ideal correlation, i.e. no cross correlations.gain (
float
) – Linear power gain factor a signal experiences when being propagated over this realization. \(1.0\) by default.seed (
int
|None
) – Seed used to initialize the pseudo-random number generator.
- Raises:
ValueError – If the length of delays, power_profile and rice_factors is not identical.
ValueError – If delays are smaller than zero.
ValueError – If power factors are smaller than zero.
ValueError – If rice factors are smaller than zero.
- classmethod Deserialize(process)[source]¶
Deserialize an object’s state.
Objects cannot be deserialized directly, instead a
Factory
must be instructed to carry out the deserialization process.- Parameters:
process (
DeserializationProcess
) – The current stage of the deserialization process. This object is generated by theFactory
and provides an interface to deserialization methods supporting multiple backends.- Return type:
- Returns:
The deserialized object.
- _realize()[source]¶
Generate a new channel realzation.
Abstract subroutine of
realize
. EachChannel
is required to implement their own_realize()
method.Returns: A new channel realization.
- Return type:
- serialize(process)[source]¶
Serialize this object’s state.
Objects cannot be serialized directly, instead a
Factory
must be instructed to carry out the serialization process.- Parameters:
process (
SerializationProcess
) – The current stage of the serialization process. This object is generated by theFactory
and provides an interface to serialization methods supporting multiple backends.- Return type:
- property antenna_correlation: AntennaCorrelation | None¶
Antenna correlations.
- Returns:
Handle to the correlation model.
None
, if no model was configured and ideal correlation is assumed.
- property correlation_distance: float¶
Correlation distance in meters.
Represents the distance over which the antenna correlation is assumed to be constant.
- property delays: ndarray¶
Delays for each propagation path in seconds.
Represented by the sequence
\[\left[\tau_{1},\, \dotsc,\, \tau_{L} \right]^{\mathsf{T}} \in \mathbb{R}_{+}^{L}\]of \(L\) propagtion delays within the respective equations.
- property doppler_frequency: float¶
Doppler frequency in \(Hz\).
Represented by \(\omega\) within the respective equations.
- property los_angle: float | None¶
Line of sight doppler angle in radians.
Represented by \(\theta_{0}\) within the respective equations.
- property los_doppler_frequency: float¶
Line of sight Doppler frequency in \(Hz\).
Represented by \(\omega\) within the respective equations.
- property num_resolvable_paths: int¶
Number of dedicated propagation paths.
Represented by \(L\) within the respective equations.
- property num_sinusoids: int¶
Number of sinusoids assumed to model the fading in time-domain.
Represented by \(N\) within the respective equations.
- Raises:
ValueError – For values smaller than zero.