Multi Target¶
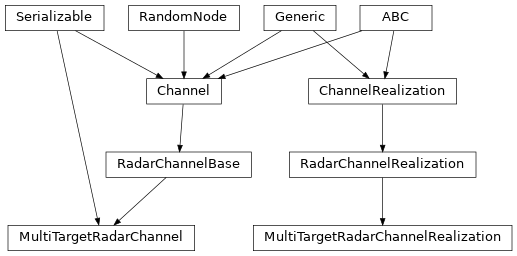
Model of a spatial radar channel featuring multiple reflecting targets.
The following minimal example outlines how to configure the channel model
within the context of a Simulation
:
- class MultiTargetRadarChannel(attenuate=True, interference=True, decorrelation_distance=inf, *args, **kwargs)[source]¶
Bases:
RadarChannelBase
[MultiTargetRadarChannelRealization
],Serializable
Model of a spatial radar channel featuring multiple reflecting targets.
- Parameters:
attenuate (bool, optional) – Should the propagated signal be attenuated during propagation modeling? Enabled by default.
interference (bool, optional) – Should the channel model consider interference between the linked devices? Enabled by default.
decorrelation_distance (float, optional) – Distance at which the channel’s random variable realizations are considered uncorrelated. \(\infty\) by default, meaning the channel is static in space.
- _realize()[source]¶
Generate a new channel realzation.
Abstract subroutine of
realize
. EachChannel
is required to implement their own_realize()
method.Returns: A new channel realization.
- Return type:
- add_target(target)[source]¶
Add a new target to the radar channel.
- Parameters:
target (RadarTarget) – Target to be added.
- Return type:
- make_target(moveable, cross_section, *args, **kwargs)[source]¶
Declare a moveable to be a target within the radar channel.
- Parameters:
moveable (Moveable) – Moveable to be declared as a target.
cross_section (RadarCrossSectionModel) – Radar cross section model of the target.
*args – Additional positional arguments passed to the target’s constructor.
**kwargs – Additional keyword arguments passed to the target’s constructor.
- Returns:
The newly created target.
- Return type:
- recall_realization(group)[source]¶
Recall a realization of this channel type from its HDF serialization.
- Parameters:
group (h5py.Group) – HDF group to which the channel realization was serialized.
- Return type:
Returns: The recalled realization instance.
- property decorrelation_distance: float¶
Decorrelation distance of the radar channel.
- Raises:
ValueError – For decorrelation distances smaller than zero.
- interfernce: bool¶
Consider interference between linked devices.
Only applies in the bistatic case, where transmitter and receiver are two dedicated device instances.
- property targets: Set[RadarTarget]¶
Set of targets considered within the radar channel.
- class MultiTargetRadarChannelRealization(consistent_realization, phase_variable, targets, interference, attenuate, sample_hooks, gain)[source]¶
Bases:
RadarChannelRealization
Realization of a spatial multi target radar channel.
Generated by the
realize
method ofMultiTargetRadarChannel
._summary_
- Parameters:
sample_hooks (Set[ChannelSampleHook[CST]], optional) – Hooks to be called after the channel is sampled.
gain (float) – Linear power gain factor a signal experiences when being propagated over this realization.
- class RadarTarget(static=False)[source]¶
Bases:
ABC
Abstract base class of radar targets.
Radar targets represent reflectors of electromagnetic waves within
RadarChannelBase
instances.- Parameters:
static (bool, optional) – Is the target visible during null hypothesis testing? Disabled by default.
- abstract sample_cross_section(impinging_direction, emerging_direction)[source]¶
Query the target’s radar cross section.
The target radr cross section is denoted by the vector \(\sigma_{\ell}\) within the respective equations.
- Parameters:
- Return type:
Returns: The assumed radar cross section in \(m^2\).
- class VirtualRadarTarget(cross_section, trajectory=None, static=False)[source]¶
Bases:
Moveable
,RadarTarget
,Serializable
Model of a spatial radar target only existing within a channe link.
- Parameters:
cross_section (RadarCrossSectionModel) – The assumed cross section model.
trajectory (Trajectory, optional) – The assumed trajectory of the target. By default, the target is assumed to be static.
static (bool, optional) – See
RadarTarget.static()
. Disabled by default.
- sample_cross_section(impinging_direction, emerging_direction)[source]¶
Query the target’s radar cross section.
The target radr cross section is denoted by the vector \(\sigma_{\ell}\) within the respective equations.
- Parameters:
- Return type:
Returns: The assumed radar cross section in \(m^2\).
- sample_trajectory(timestamp)[source]¶
Sample the target’s trajectory at a given time.
- Parameters:
timestamp (float) – Time at which to sample the trajectory in seconds.
- Return type:
Returns: A sample of the trajectory.
- property cross_section: RadarCrossSectionModel¶
The represented radar cross section model.
- class PhysicalRadarTarget(cross_section, moveable, static=False)[source]¶
Bases:
RadarTarget
,Serializable
Model of a spatial radar target representing a moveable object.
The radar target will always be modeled at its moveable global position.
- Parameters:
cross_section (RadarCrossSectionModel) – The assumed cross section model.
moveable (Moveable) – The moveable object this radar target represents.
static (bool, optional) – See
RadarTarget.static()
. Disabled by default.
- sample_cross_section(impinging_direction, emerging_direction)[source]¶
Query the target’s radar cross section.
The target radr cross section is denoted by the vector \(\sigma_{\ell}\) within the respective equations.
- Parameters:
- Return type:
Returns: The assumed radar cross section in \(m^2\).
- sample_trajectory(timestamp)[source]¶
Sample the target’s trajectory at a given time.
- Parameters:
timestamp (float) – Time at which to sample the trajectory in seconds.
- Return type:
Returns: A sample of the trajectory.
- property cross_section: RadarCrossSectionModel¶
The represented radar cross section model.
- class RadarCrossSectionModel[source]¶
Bases:
ABC
Base class for spatial radar cross section models.
- class FixedCrossSection(cross_section)[source]¶
Bases:
RadarCrossSectionModel
Model of a fixed cross section.
Can be interpreted as a spherical target floating in space.
- Parameters:
cross_section (float) – The cross section in \(\mathrm{m}^2\).
- get_cross_section(_, __)[source]¶
Query the model’s cross section.
- Parameters:
- Return type:
Returns: The assumed cross section in \(m^2\).
- property cross_section: float¶
The assumed cross section.
Returns: The cross section in \(\mathrm{m}^2\).
- Raises:
ValueError – For cross sections smaller than zero.