Single Carrier¶
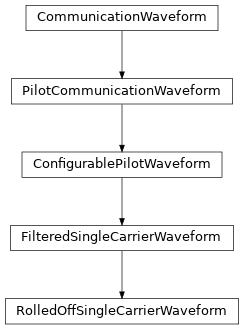
Single carrier waveforms modulate only a single discrete carrier frequency. They are typically applying a shaping filter around the modulated frequency, of which the following are currently available:
- class FilteredSingleCarrierWaveform(symbol_rate, num_preamble_symbols, num_data_symbols, num_postamble_symbols=0, pilot_rate=0, guard_interval=0.0, oversampling_factor=4, pilot_symbol_sequence=None, repeat_pilot_symbol_sequence=True, **kwargs)[source]¶
Bases:
ConfigurablePilotWaveform
This method provides a class for a generic PSK/QAM modem.
The modem has the following characteristics: - root-raised cosine filter with arbitrary roll-off factor - arbitrary constellation, as defined in modem.tools.psk_qam_mapping:PskQamMapping
This implementation has currently the following limitations: - hard output only (no LLR) - no reference signal - ideal channel estimation - equalization of ISI with FMCW in AWGN channel only - no equalization (only amplitude and phase of first propagation path is compensated)
- Parameters:
symbol_rate (float) – Rate at which symbols are being generated in Hz.
num_preamble_symbols (int) – Number of preamble symbols within a single communication frame.
num_data_symbols (int) – Number of data symbols within a single communication frame.
num_postamble_symbols (int, optional) – Number of postamble symbols within a single communication frame.
guard_interval (float, optional) – Guard interval between communication frames in seconds. Zero by default.
oversampling_factor (int, optional) – The oversampling factor of the waform.
pilot_rate (int, optional) – Pilot symbol rate. Zero by default, i.e. no pilot symbols.
pilot_symbol_sequence (Optional[PilotSymbolSequence], optional) – The configured pilot symbol sequence. Uniform by default.
repeat_pilot_symbol_sequence (bool, optional) – Allow the repetition of pilot symbol sequences. Enabled by default.
kwargs (Any) – Waveform generator base class initialization parameters.
- demodulate(signal)[source]¶
Demodulate a base-band signal stream to data symbols.
- Parameters:
signal (numpy.ndarray) – Vector of complex-valued base-band samples of a single communication frame.
- Return type:
- Returns:
The demodulated communication symbols
- map(bits)[source]¶
Map a stream of bits to data symbols.
- Parameters:
data_bits (numpy.ndarray) – Vector containing a sequence of L hard data bits to be mapped onto data symbols.
- Returns:
Mapped data symbols.
- Return type:
- modulate(symbols)[source]¶
Modulate a stream of data symbols to a base-band signal containing a single data frame.
- Parameters:
data_symbols (Symbols) – Singular stream of data symbols to be modulated by this waveform.
- Return type:
Returns: Samples of the modulated base-band signal.
- pick(symbols)[source]¶
Pick the mapped symbols from the communicaton frame.
Additionally removes interleaved pilot symbols.
- Parameters:
placed_symbols (StatedSymbols) – The placed symbols.
- Return type:
Returns: The symbols with the mapped symbols picked from the frame.
- place(data_symbols)[source]¶
Place the mapped symbols within the communicaton frame.
Additionally interleaves pilot symbols.
Returns: The symbols with the mapped symbols placed within the frame.
- plot_filter()[source]¶
Plot the transmit filter shape.
- Return type:
- Returns:
Handle to the generated matplotlib figure.
- plot_filter_correlation()[source]¶
Plot the convolution between transmit and receive filter shapes.
- Return type:
- Returns:
Handle to the generated matplotlib figure.
- unmap(symbols)[source]¶
Map a stream of data symbols to data bits.
- Parameters:
symbols (Symbols) – Sequence of K data symbols to be mapped onto bit sequences.
- Returns:
Vector containing the resulting sequence of L data bits In general, L is greater or equal to K.
- Return type:
np.ndarray
- property bit_energy: float¶
Returns the theoretical average (discrete-time) bit energy of the modulated baseband_signal.
Energy of baseband_signal \(x[k]\) is defined as \(\sum{|x[k]}^2\) Only data bits are considered, i.e., reference, guard intervals are ignored.
- property modulation_order: int¶
Access the modulation order.
- Returns:
The modulation order.
- Return type:
- property num_data_symbols: int¶
Number of data symbols per frame.
- Raises:
ValueError – If num is smaller than zero.
- property num_guard_samples: int¶
Number of samples within the guarding section of a frame.
- Returns:
Number of samples.
- Return type:
- property num_postamble_symbols: int¶
Number of postamble symbols.
Transmitted at the end of communication frames.
Returns: The number of symbols.
- Raises:
ValueError – If the number of symbols is smaller than zero.
- property num_preamble_symbols: int¶
Number of preamble symbols.
Transmitted at the beginning of communication frames.
Returns: The number of symbols.
- Raises:
ValueError – If the number of symbols is smaller than zero.
- property pilot_rate: int¶
Repetition rate of pilot symbols within the frame.
A pilot rate of zero indicates no pilot symbols within the data frame.
- Returns:
Rate in number of symbols
- Raises:
ValueError – If the pilot rate is smaller than zero.
- property pilot_signal: Signal¶
Model of the pilot sequence within this communication waveform.
- Returns:
The pilot sequence.
- Return type:
- property power: float¶
Returns the theoretical average symbol (unitless) power,
Power of baseband_signal \(x[k]\) is defined as \(\sum_{k=1}^N{|x[k]|}^2 / N\) Power is the average power of the data part of the transmitted frame, i.e., bit energy x raw bit rate
- property sampling_rate: float¶
Rate at which the waveform generator signal is internally sampled.
- Returns:
Sampling rate in Hz.
- Return type:
- property symbol_energy: float¶
The theoretical average symbol (discrete-time) energy of the modulated baseband_signal.
Energy of baseband_signal \(x[k]\) is defined as \(\sum{|x[k]}^2\) Only data bits are considered, i.e., reference, guard intervals are ignored.
- Returns:
The average symbol energy in UNIT.
- property symbol_rate: float¶
Repetition rate of symbols.
- Returns:
Symbol rate in Hz.
- Raises:
ValueError – For rates smaller or equal to zero.
- class RolledOffSingleCarrierWaveform(relative_bandwidth=1.0, roll_off=0.0, filter_length=16, *args, **kwargs)[source]¶
Bases:
FilteredSingleCarrierWaveform
Base class for single carrier waveforms applying linear filters longer than a single symbol duration.
- Parameters:
relative_bandwidth (float, optional) – Bandwidth relative to the configured symbol rate. One by default, meaning the pulse bandwidth is equal to the symbol rate in Hz, assuming zero roll_off.
roll_off (float, optional) – Filter pulse shape roll off factor between zero and one. Zero by default, meaning no inter-symbol interference at the sampling instances.
filter_length (float, optional) – Filter length in modulation symbols. 16 by default.
- property bandwidth: float¶
Bandwidth of the frame generated by this generator.
Used to estimate the minimal sampling frequency required to adequately simulate the scenario.
- Returns:
Bandwidth in Hz.
- Return type:
- property filter_length: int¶
Filter length in modulation symbols.
Configures how far the shaping filter stretches in terms of the number of modulation symbols it overlaps with.
- Returns:
Filter length in number of modulation symbols.
- Raises:
ValueError – For filter lengths smaller than one.
- property relative_bandwidth: float¶
Bandwidth relative to the configured symbol rate.
- Raises:
ValueError – On values smaller or equal to zero.
- property roll_off: float¶
Filter pulse shape roll off factor.
- Raises:
ValueError – On values smaller than zero or larger than one.