Space-Time Block Codes¶
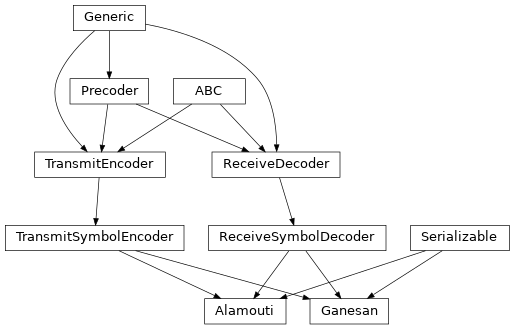
Space-Time Block codes distribute communication symbols over multiple antennas and time slots.
They can be configured by adding an instance to the
SymbolPrecoding
of a Modem
exposed by the precoding
attribute.
The following example shows how to configure a modem with a Alamouti precoder within a \(2\times 2\) MIMO communication link:
1# Create a new simulation featuring a 2x2 MIMO link between two devices
2simulation = Simulation()
3tx_device = simulation.new_device(
4 antennas=SimulatedUniformArray(SimulatedIdealAntenna(AntennaMode.TX), 0.1, (2,)),
5)
6
7rx_device = simulation.new_device(
8 antennas=SimulatedUniformArray(SimulatedIdealAntenna(AntennaMode.RX), 0.1, (1,)),
9)
10
11# Create a link between the two devices
12link = SimplexLink()
13link.connect(tx_device, rx_device)
14
15# Configure a single carrier waveform
16waveform = RootRaisedCosineWaveform(
17 oversampling_factor=4,
18 symbol_rate=1e6,
19 num_preamble_symbols=16,
20 num_data_symbols=32,
21 modulation_order=64,
22 roll_off=.9,
23 channel_estimation=SingleCarrierIdealChannelEstimation(simulation.scenario.channel(tx_device, rx_device), tx_device, rx_device),
24)
25link.waveform = waveform
26
27# Configure the precoding
28link.transmit_symbol_coding[0] = Alamouti()
29link.receive_symbol_coding[0] = Alamouti()
Note that Alamouti precoding requires channel state information at the receiver,
therefore waveform’s channel_estimation
attribute must be configured.
The following example shows how to configure a modem with a Ganesan precoder within a \(4\times 4\) MIMO communication link:
1# Create a new simulation featuring a 4x4 MIMO link between two devices
2simulation = Simulation()
3tx_device = simulation.new_device(
4 antennas=SimulatedUniformArray(SimulatedIdealAntenna(AntennaMode.TX), 0.1, (4,)),
5)
6
7rx_device = simulation.new_device(
8 antennas=SimulatedUniformArray(SimulatedIdealAntenna(AntennaMode.RX), 0.1, (4,)),
9)
10
11# Create a link between the two devices
12link = SimplexLink()
13link.connect(tx_device, rx_device)
14
15# Configure a single carrier waveform
16waveform = RootRaisedCosineWaveform(
17 oversampling_factor=4,
18 symbol_rate=1e6,
19 num_preamble_symbols=16,
20 num_data_symbols=32,
21 modulation_order=64,
22 roll_off=.9,
23 channel_estimation=SingleCarrierIdealChannelEstimation(simulation.scenario.channel(tx_device, rx_device), tx_device, rx_device),
24)
25link.waveform = waveform
26
27# Configure the precoding
28link.transmit_symbol_coding[0] = Ganesan()
29link.receive_symbol_coding[0] = Ganesan()
Note that Ganesan precoding requires channel state information at the receiver,
therefore waveform’s channel_estimation
attribute must be configured.
- class Alamouti[source]¶
Bases:
TransmitSymbolEncoder
,ReceiveSymbolDecoder
,Serializable
Alamouti precoder distributing symbols in space and time.
Support for 2 transmit antennas only. Refer to Alamouti[1] for further information.
- decode_symbols(symbols, num_output_streams)[source]¶
Decode data for STBC with 2 antenna streams
Received signal with equal noise power is assumed, the decoded signal has same noise level as input.
- Parameters:
symbols (StatedSymbols) – Input signal with \(N \times K\) symbol blocks.
num_output_streams (int) –
- Number of required streams resulting from the decoding process.
Will be ignored by this decoder.
- Return type:
Returns: Decoded data with size \(N \times K\)
- encode_symbols(symbols, num_output_streams)[source]¶
Encode data into multiple antennas with space-time/frequency block codes.
- Parameters:
symbols (StatedSymbols) – Input signal featuring \(K\) blocks.
num_output_streams (int) – Number of required streams resulting from the encoding process. Should always be 2 for Alamouti encoding.
- Return type:
Returns: Encoded data with size \(2 \times K\) symbols
- Raises:
ValueError – If more than a single symbol stream is provided.
ValueError – If the number of transmit antennas is not two.
ValueError – If the number of data symbols is not even.
- class Ganesan[source]¶
Bases:
TransmitSymbolEncoder
,ReceiveSymbolDecoder
,Serializable
Girish Ganesan and Petre Stoica general precoder distributing symbols in space and time.
Supports 4 transmit antennas. Features a \(\frac{3}{4}\) symbol rate. Refer to Ganesan and Stoica[2] for further information.
- decode_symbols(symbols, num_output_streams)[source]¶
Decode data for STBC with 4 antenna streams Note that Ganesan schema’s symbol rate is \(\frac{3}{4}\) so the decoding process decreases the number of blocks by \(\frac{3}{4}\).
- Parameters:
symbols (StatedSymbols) – Input signal with \(4 \times N\) symbol blocks.
num_output_streams (int) – Number of required streams resulting from the decoding process. Will be ignored by this decoder.
- Return type:
- Returns:
Decoded data with size \(3 \times N\) Returned channel states are initialized with ones (np.ones is used).
- encode_symbols(symbols, num_output_streams)[source]¶
Encode data into multiple antennas with space-time/frequency block codes. Note that Ganesan schema’s symbol rate is \(\frac{3}{4}\) so the encoding process increases the number of blocks by \(\frac{4}{3}\).
- Parameters:
symbols (StatedSymbols) – Input signal featuring \(K\) blocks.
num_output_streams (int) – Number of required streams resulting from the encoding process. Should always be 4 for Ganesan encoding.
- Return type:
- Returns:
Encoded data with size \(\frac{4}{3} \times K\) symbol blocks. Thus num_blocks is changed to num_blocks / 3 * 4. Returned channel states are initialized with ones (np.ones is used).
- Raises:
ValueError – If more than a single symbol stream is provided.
RuntimeError – If the number of transmit antennas is not four.
ValueError – If the number of data symbols blocks is not divisable by three.