Duplex Modem¶
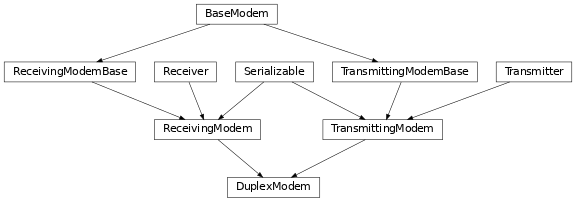
Duplex modems represent the signal processing chain of a communication device simulatenously transmitting and receiving information, implementing the digital signal processing before digital-to-analog conversion and after analog-to-digital conversion, respectively. They are a combination of a Transmitting Modem and a Receiving Modem.
After a DuplexModem
is added as a
type of Transmitter
to a Device
,
a call to Device.transmit
will be delegated
to TransmittingModem._transmit()
.
Similarly, after a DuplexModem
is added as a
type of Receiver
to a Device
,
a call to Device.receive
will be delegated
to ReceivingModem._receive()
1# Initialize a new simulation considering a single device
2simulation = Simulation()
3device = simulation.new_device(carrier_frequency=1e10)
4
5# Configure the link modeling the devices' transmit DSP
6modem = DuplexModem()
7device.add_dsp(modem)
8
9# Configure the links's waveform
10waveform = RootRaisedCosineWaveform(
11 oversampling_factor=4,
12 symbol_rate=1e6,
13 num_preamble_symbols=16,
14 num_data_symbols=32,
15 modulation_order=4,
16)
17modem.waveform = waveform
For a detailed description of the transmit and receive routines, refer to the Transmitting Modem and a Receiving Modem of the base classes.
The barebone configuration can be extend by additional components such as
Custom Bit Sources
,
Synchronization
,
Stream Precoding
,
Channel Estimation
,
Symbol Precoding
,
Channel Equalization
and
Bit Encoders
:
1# Configure a custom bits source for the modem
2modem.bits_source = RandomBitsSource(seed=42)
3
4# Configure the waveform's synchronization routine
5modem.waveform.synchronization = SingleCarrierCorrelationSynchronization()
6
7# Add a custom stream precoding to the modem
8modem.transmit_signal_coding[0] = ConventionalBeamformer()
9modem.receive_signal_coding[0] = ConventionalBeamformer()
10
11# Add a custom symbol precoding to the modem
12modem.transmit_symbol_coding[0] = DFT()
13modem.receive_symbol_coding[0] = DFT()
14
15# Configure the waveform's channel estimation routine
16modem.waveform.channel_estimation = SingleCarrierLeastSquaresChannelEstimation()
17
18# Configure the waveform's channel equalization routine
19modem.waveform.channel_equalization = SingleCarrierZeroForcingChannelEqualization()
20
21# Add forward error correction encodings to the transmitted bit stream
22modem.encoder_manager.add_encoder(RepetitionEncoder(32, 3))
23modem.encoder_manager.add_encoder(BlockInterleaver(192, 32))
- class DuplexModem(*args, bits_source=None, selected_transmit_ports=None, selected_receive_ports=None, **kwargs)[source]¶
Bases:
TransmittingModem
,ReceivingModem
Representation of a wireless modem simultaneously transmitting and receiving.
- Parameters:
bits_source (BitsSource, optional) – Source configuration of communication bits transmitted by this modem. Bits are randomly generated by default.
selected_transmit_ports (Sequence[int] | None) – Indices of antenna ports selected for transmission from the operated
Device's
antenna array. If not specified, all available ports will be considered.selected_receive_ports (Sequence[int] | None) – Indices of antenna ports selected for reception from the operated
Device's
antenna array. If not specified, all available ports will be considered.*args – Modem initialization parameters. Refer to
TransmittingModem
andReceivingModem
for further details.**kwargs – Modem initialization parameters. Refer to
TransmittingModem
andReceivingModem
for further details.
- yaml_tag: Optional[str] = 'Modem'¶
YAML serialization tag