Single Carrier Decoding¶
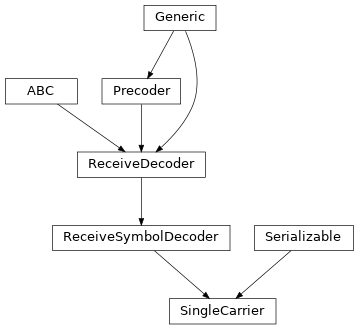
Single carrier decoding combines individually demodulated symbols from multiple antenna streams into a single stream of symbols, given that they have been transmitted by a single-antenna device.
It can be configured by adding an instance to the
SymbolPrecoding
of a Modem
exposed by the precoding
attribute:
1# Create a new simulation featuring a 1x2 SIMO link between two devices
2simulation = Simulation()
3tx_device = simulation.new_device()
4rx_device = simulation.new_device(
5 antennas=SimulatedUniformArray(SimulatedIdealAntenna(AntennaMode.RX), 0.1, (2,)),
6)
7
8# Create a link between the two devices
9link = SimplexLink()
10link.connect(tx_device, rx_device)
11
12# Configure a single carrier waveform
13waveform = RootRaisedCosineWaveform(
14 oversampling_factor=4,
15 symbol_rate=1e6,
16 num_preamble_symbols=16,
17 num_data_symbols=32,
18 modulation_order=64,
19 roll_off=.9,
20 channel_estimation=SingleCarrierIdealChannelEstimation(simulation.scenario.channel(tx_device, rx_device), tx_device, rx_device),
21)
22link.waveform = waveform
23
24# Configure the precoding
25link.receive_symbol_coding[0] = SingleCarrier()
Note that decoding requires channel state information at the receiver,
therefore waveform’s channel_estimation
attribute must be configured.
- class SingleCarrier[source]¶
Bases:
ReceiveSymbolDecoder
,Serializable
Single Carrier data symbol precoding step.
Takes a on-dimensional input stream and distributes the symbols to multiple output streams.
- decode_symbols(symbols, num_output_streams)[source]¶
Decode a data stream before reception.
This operation may modify the number of streams as well as the number of data symbols per stream.
- Parameters:
symbols (StatedSymbols) – Symbols to be decoded.
num_output_streams (int) – Number of required output streams after decoding.
- Return type:
Returns: Decoded symbols.