Simulation¶
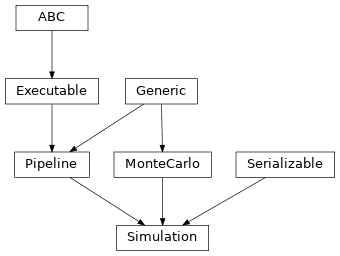
The Simulation
class extends
HermesPy’s core distributed MonteCarlo
simulation framework to support parameterized physical layer simulations of wireless
communication and sensing scenarios.
It manages the configuration of the physical layer description represented by
SimulationScenarios
.
Once the user has configured the physical layer description,
a call to run
will launch a full
Monte Carlo simulation iterating over the configured parameter grid.
During simulation runtime, the follwing sequence of simulation stages is repeatedly executed:
Generate digital base-band signal models to be transmitted by the simulated devices
Simulate the hardware effects of devices during transmission
Realize all channel models
Propagate the device transmissions over the channel realizations
Simulate the hardware effects of devices during reception
Digitally process base-band signal models received by the simulated devices
Evaluate the configured performance metrics
- class Simulation(scenario=None, num_samples=100, drop_duration=0.0, drop_interval=inf, plot_results=False, dump_results=True, console_mode=ConsoleMode.INTERACTIVE, ray_address=None, results_dir=None, verbosity=Verbosity.INFO, seed=None, num_actors=None)[source]¶
Bases:
Serializable
,Pipeline
[SimulationScenario
,SimulatedDevice
],MonteCarlo
[SimulationScenario
]Executable HermesPy simulation configuration.
- Parameters:
scenario (SimulationScenario, optional) – The simulated scenario. If none is provided, an empty one will be initialized.
num_samples (int, optional) – Number of drops generated per sweeping grid section. 100 by default.
drop_duration (float, optional) – Duration of simulation drops in seconds.
drop_interval (float, optional) – Interval at which drops are being generated in seconds. If not specified, only a single drop is generated at the beginning of the simulation.
plot_results (bool, optional) – Plot results after simulation runs. Disabled by default.
dump_results (bool, optional) – Dump results to files after simulation runs. Enabled by default.
console_mode (ConsoleMode, optional) – Console output behaviour during execution.
ray_address (str, optional) – The address of the ray head node. If None is provided, the head node will be launched in this machine.
results_dir (str, optional) – Directory in which all simulation artifacts will be dropped.
verbosity (Union[str, Verbosity], optional) – Information output behaviour during execution.
seed (int, optional) – Random seed used to initialize the pseudo-random number generator.
num_actors (int, optional) – Number of actors to be deployed for parallel execution. If None is provided, the number of actors will be set to the number of available CPU cores.
- classmethod from_yaml(constructor, node)[source]¶
Recall a new Simulation instance from YAML.
- Parameters:
constructor (SafeConstructor) – A handle to the constructor extracting the YAML information.
node (Node) – YAML node representing the Simulation serialization.
- Returns:
Newly created Simulation instance.
- Return type:
- set_channel(alpha, beta, channel)[source]¶
Specify a channel within the channel matrix.
Convenience method resolving to the
set_channel
method of the managedSimulationScenario
instance, which can be accessed via thescenario
property.- Parameters:
receiver (SimulatedDevice) – Index of the receiver within the channel matrix.
transmitter (SimulatedDevice) – Index of the transmitter within the channel matrix.
channel (Channel | None) – The channel instance to be set at position (transmitter_index, receiver_index).
- Return type:
- classmethod to_yaml(representer, node)[source]¶
Serialize an Simulation object to YAML.
- Parameters:
representer (SafeRepresenter) – A handle to a representer used to generate valid YAML code. The representer gets passed down the serialization tree to each node.
node (Simulation) – The Simulation instance to be serialized.
- Returns:
The serialized YAML node
- Return type:
Node
- property console: Console¶
Console the Simulation writes to.
- Returns:
Handle to the console.
- Return type:
Console
- property console_mode: ConsoleMode¶
Console mode during runtime.
Returms: The current console mode.
- property drop_interval: float¶
Interval at which drops are being generated in seconds.
- Raises:
ValueError for values smaller or equal to zero. –
- dump_results: bool¶
Dump results to files after simulation runs.
- property num_samples: int¶
Number of samples per simulation grid element.
- Returns:
Number of samples
- Return type:
- Raises:
ValueError – If number of samples is smaller than one.
- plot_results: bool¶
Plot results after simulation runs