Transmitting Modem¶
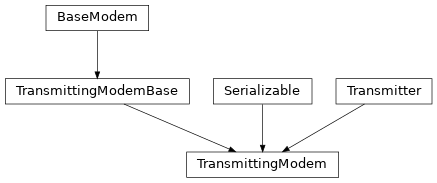
Transmitting modems represent the digital signal processing operations performed within a communication system up to the point of digital-to-analog conversion.
After a TransmittingModem
is added as a
type of Transmitter
to a Device
,
a call to Device.transmit
will be delegated
to TransmittingModem._transmit()
:
Initially, the TransmittingModem
configured BitsSource
will be queried for a sequence of data bits required to moulate a single frame.
The specic number of bits depends several factors, primarily the Waveform
configured by the respective
waveform
, the precodings and the forward error correction.
The sequence of data bits is subseuently encoded for forward error correction by the EncoderManager
configured by the respective
encoder_manager
.
The resulting sequence of encoded bits is then mapped to a sequence of communication symbols by the CommunicationWaveform's
map
method.
In the following step, the sequence of communication symbols is precoded by the SymbolPrecoding
configured by the respective
precoding
property.
Finally, a set of baseband streams is generate by placing
and modulating
the precoded communication symbols.
The baseband streams are then encoded by the TransmitStreamCoding
.
This sequence of steps can be repeated multiple times to generate a sequence of communication frames, if required.
Note that, as a bare minimum, only the waveform
has to be configured for a fully functional TransmittingModem
.
The following snippet shows how to configure a TransmittingModem
with a RootRaisedCosineWaveform
wavform implementing a
CommunicationWaveform
:
1
2# Initialize a new simulation considering a single device
3simulation = Simulation()
4device = simulation.new_device(carrier_frequency=1e10)
5
6# Configure the modem modeling the device's transmit DSP
7tx_modem = TransmittingModem(device=device)
8
9# Configure the modem's waveform
10waveform = RootRaisedCosineWaveform(
11 oversampling_factor=4,
12 symbol_rate=1e6,
13 num_preamble_symbols=16,
14 num_data_symbols=32,
15 modulation_order=64,
16)
17tx_modem.waveform = waveform
This barebone configuration can be extended by adding additional components such as
BitsSources
, Bit Encoders
,
Symbol Precoders
and
Stream Precoders
:
1# Add a custom bits source to the modem
2tx_modem.bits_source = RandomBitsSource(seed=42)
3
4# Add forward error correction encodings to the transmitted bit stream
5tx_modem.encoder_manager.add_encoder(RepetitionEncoder(32, 3))
6tx_modem.encoder_manager.add_encoder(BlockInterleaver(192, 32))
7
8# Add a custom symbol precoding to the modem
9tx_modem.precoding[0] = DFT()
10
11# Add a custom stream precoding to the modem
12tx_modem.transmit_stream_coding[0] = ConventionalBeamformer()
- class TransmittingModem(*args, device=None, selected_transmit_ports=None, **kwargs)[source]¶
Bases:
TransmittingModemBase
[CommunicationWaveform
],Transmitter
[CommunicationTransmission
],Serializable
Representation of a wireless modem exclusively transmitting.
- Parameters:
device (Device, optional) – Device operated by the modem.
selected_transmit_ports (Sequence[int] | None) – Indices of antenna ports selected for transmission from the operated
Device's
antenna array. If not specified, all available ports will be considered.*args – Modem initialization parameters. Refer to
TransmittingModemBase
for further details.**kwargs – Modem initialization parameters. Refer to
TransmittingModemBase
for further details.
- property device: Device | None¶
Device this object is assigned to.
None
if this object is currently considered floating / unassigned.
- property power: float¶
Expected power of the transmitted signal in Watts.
Note
Applies only to the signal-carrying parts of the transmission, silent parts shuch as guard intervals should not be considered.
- class TransmittingModemBase(bits_source=None, *args, **kwargs)[source]¶
Bases:
Generic
[CWT
],BaseModem
[CWT
]Base class of signal processing algorithms transmitting information.
- Parameters:
bits_source (BitsSource, optional) – Source configuration of communication bits transmitted by this modem. Bits are randomly generated by default.
- _transmit(duration=-1.0)[source]¶
Returns an array with the complex base-band samples of a waveform generator.
The signal may be distorted by RF impairments.
- Parameters:
duration (float, optional) – Length of signal in seconds.
- Return type:
- Returns:
Transmitted information.
- property bits_source: BitsSource¶
Source configuration of communication transmitted by this modem.
Returns: A handle to the source configuration.
- property transmit_stream_coding: TransmitStreamCoding¶
Stream MIMO coding configuration during signal transmission.
Returns: Handle to the coding configuration.