Radar¶
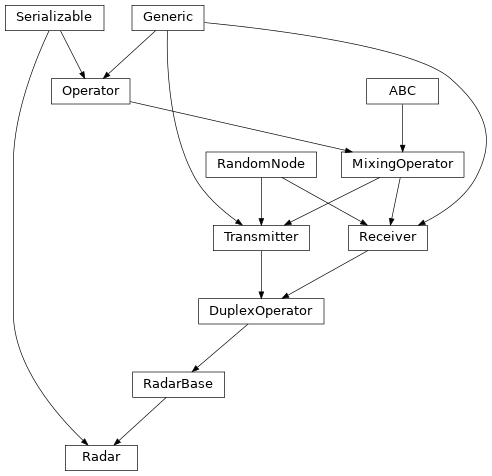
- class Radar(device=None, seed=None)[source]¶
Bases:
RadarBase
[RadarTransmission
,RadarReception
],Serializable
Signal processing pipeline of a monostatic radar sensing its environment.
The radar can be configured by assigning four composite objects to respective property attributes:
Property
Type
Of those, only a
RadarWaveform
is mandatory. Beamformers, i.e. aTransmitBeamformer
and aReceiveBeamformer
, are only required when the radar is assigned to aDevice
configured to multipleantennas
. ARadarDetector
is optional, if not configured the radar’s generatedRadarReception
will not contain aRadarPointCloud
.When assigned to a
Device
, device transmission will trigger the radar to generate aRadarTransmission
by executing the following sequence of calls:sequenceDiagram participant Device participant Radar participant RadarWaveform participant TransmitBeamformer Device ->> Radar: _transmit() Radar ->> RadarWaveform: ping() RadarWaveform -->> Radar: Signal Radar ->> TransmitBeamformer: transmit(Signal) TransmitBeamformer -->> Radar: Signal Radar -->> Device: RadarTransmissionInitially, the
ping
method of theRadarWaveform
is called to generate the model of a single-antenna radar frame. ForDevices
configured to multipleantennas
, the configuredTransmitBeamformer
is called to encode the signal for each antenna. The resulting multi-antenna frame, contained within the returnRadarTransmission
, is cached at the assignedDevice
.When assigned to a
Device
, device reception will trigger the radar to generate aRadarReception
by executing the following sequence of calls:sequenceDiagram participant Device participant Radar participant ReceiveBeamformer participant RadarWaveform participant RadarDetector Device ->> Radar: _receive(Signal) Radar ->> ReceiveBeamformer: probe(Signal) ReceiveBeamformer -->> Radar: line_signals loop Radar ->> RadarWaveform: estimate(line_signal) RadarWaveform -->> Radar: line_estimate end Radar ->> RadarDetector: detect(line_estimates) RadarDetector -->> Radar: RadarPointCloud Radar -->> Device: RadarReceptionInitially, the
probe
method of theReceiveBeamformer
is called to generate a sequence of line signals from each direction of interest. We refer to them as line signals as they are the result of an antenna arrays beamforing towards a single direction of interest, so the signal can be though of as having propagated along a single line pointing towards the direction of interest. This step is only executed forDevices
configured to multipleantennas
. The sequence of line signals are then indiviually processed by theestimate
method of theRadarWaveform
, resulting in a line estimate representing a range-doppler map for each direction of interest. This sequence line estimates is combined to a singleRadarCube
. If aRadarDetector
is configured, thedetect
method is called to generate aRadarPointCloud
from theRadarCube
. The resulting information is cached as aRadarReception
at the assignedDevice
.- Parameters:
- property frame_duration: float¶
Duration of a single sample frame in seconds.
Denoted as \(T_{\mathrm{F}}\) of unit \(\left[ T_{\mathrm{F}} \right] = \mathrm{s}\) in literature.
- property max_range: float¶
The radar’s maximum detectable range in meters.
Denoted by \(R_{\mathrm{Max}}\) of unit \(\left[ R_{\mathrm{Max}} \right] = \mathrm{m}\) in literature. Convenience property that resolves to the configured
RadarWaveform
’smax_range
property. Returns \(R_{\mathrm{Max}} = 0\) if no waveform is configured.
- property power: float¶
Expected power of the transmitted signal in Watts.
Note
Applies only to the signal-carrying parts of the transmission, silent parts shuch as guard intervals should not be considered.
- property sampling_rate: float¶
The operator’s preferred sampling rate in Hz.
Denoted as \(f_{\mathrm{S}}\) of unit \(\left[ f_{\mathrm{S}} \right] = \mathrm{Hz} = \tfrac{1}{\mathrm{s}}\) in literature.
- property velocity_resolution: float¶
The radar’s velocity resolution in meters per second.
Denoted by \(\Delta v\) of unit \(\left[ \Delta v \right] = \frac{\mathrm{m}}{\mathrm{s}}\) in literature. Computed as
\[\Delta v = \frac{c_0}{f_{\mathrm{c}}} \Delta f_{\mathrm{Res}}\]querying the configured
RadarWaveform
’srelative_doppler_resolution
property \(\Delta f_{\mathrm{Res}}\).
- property waveform: RadarWaveform | None¶
Description of the waveform to be transmitted and received by this radar.
None
if no waveform is configured.During
transmit
/_transmit
, theRadarWaveform
’sping()
method is called to generate a signal to be transmitted by the radar. Duringreceive
/_receive
, theRadarWaveform
’sestimate()
method is called multiple times to generate range-doppler line estimates for each direction of interest.
- class RadarBase(*args, **kwargs)[source]¶
Bases:
Generic
[RTT
,RRT
],DuplexOperator
[RTT
,RRT
]Base class class for radar sensing signal processing pipelines.
- Parameters:
device (Device, optional) – Device the duplex operator operates.
- property detector: RadarDetector | None¶
Detector routine configured to generate point clouds from radar cubes.
If configured, during
_receive
/receive
, the detector’sdetect
method is called to generate aRadarPointCloud
. If not configured, i.e.None
, the generatedRadarReception
’scloud
property will beNone
.
- property receive_beamformer: ReceiveBeamformer | None¶
Beamforming applied during signal reception.
The
TransmitBeamformer
’sreceive
method is called as a subroutine ofReceiver.receive()
. Configuration is required for if the assignedDevice
features multipleantennas
.
- property transmit_beamformer: TransmitBeamformer | None¶
Beamforming applied during signal transmission.
The
TransmitBeamformer
’stransmit
method is called as a subroutine ofTransmitter.transmit()
. Configuration is required for if the assignedDevice
features multipleantennas
.