Simulation Scenario¶
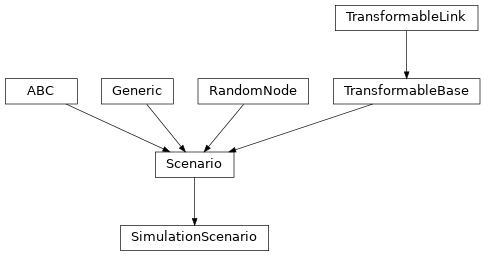
Simulation scenarios contain the complete description of a physical layer scenario to be simulated.
This includes primarily the set of physical devices and their linking channel models.
In the case of Monte Carlo simulations, they are managed by the Simulation
class
and acessible through the scenario
property.
- class SimulationScenario(default_channel=None, noise_level=None, noise_model=None, *args, **kwargs)[source]¶
Bases:
Scenario
[SimulatedDevice
,SimulatedDeviceState
,SimulatedDrop
]Description of a physical layer wireless communication scenario.
- Parameters:
default_channel (Channel, optional) – Default channel model to be assumed for all device links. If not specified, the default_channel is set to an ideal distortionless channel model.
noise_level (NoiseLevel, optional) – Global noise level of the scenario assumed for all devices. If not specified, the noise configuration is device-specific.
noise_model (NoiseModel, optional) – Global noise model of the scenario assumed for all devices. If not specified, the noise configuration is device-specific.
- add_device(device)[source]¶
Add a new device to the scenario.
- Parameters:
device (Device) – New device to be added to the scenario.
- Raises:
ValueError – If the device already exists.
RuntimeError – If the scenario is not in default mode.
- Return type:
- channel(alpha_device, beta_device)[source]¶
Access a specific channel between two devices.
- Parameters:
alpha_device (SimulatedDevice) – First device linked by the requested channel.
beta_device (SimulatedDevice) – Second device linked by the requested channel.
- Returns:
Channel between transmitter and receiver.
- Return type:
- Raises:
ValueError – Should alpha_device or beta_device not be registered with this scenario.
- device_channels(device, active_only=False)[source]¶
Collect all channels to which a specific device is linked.
- Parameters:
device (SimulatedDevice) – The device in question.
active_only (bool, optional) – Consider only active channels. A channel is considered active if its gain is greater than zero. Disabled by default, so all channels are considered.
- Return type:
Returns: A set of unique channel instances.
- Raises:
ValueError – Should device is not registered within this scenario.
- generate_outputs(transmissions, states=None, trigger_realizations=None)[source]¶
Generate signals emitted by devices.
- Parameters:
transmissions (Sequence[Sequence[Transmission]]) – Results of all transmitting DSP algorithms.
states (Sequence[SimulatedDeviceState | None], optional) – States of the transmitting devices. If not specified, the current device states will be queried by calling
Device.state()
.trigger_realizations (Sequence[TriggerRealization], optional) – Realizations of the device’s trigger models.
- Return type:
Returns: List of device outputs.
- new_device(*args, **kwargs)[source]¶
Add a new device to the simulation scenario.
- Returns:
Newly added simulated device.
- Return type:
- process_inputs(impinging_signals, states=None, trigger_realizations=None, leaking_signals=None)[source]¶
Process input signals impinging onto the scenario’s devices.
- Parameters:
impinging_signals (Sequence[DeviceInput | Signal | Sequence[Signal]] | Sequence[Sequence[Signal]]) – list of signals impinging onto the devices.
states (Sequence[SimulatedDeviceState], optional) – Sequence of simulated device states at the time of signal impingement. If not specified, the device states are assumed to be at the initial state.
trigger_realizations (Sequence[TriggerRealization], optional) – Sequence of trigger realizations. If not specified, ideal triggerings are assumed for all devices.
leaking_signals (Sequence[Signal] | Sequence[Sequence[Signal]], optional) – Signals leaking from transmit to receive chains within the individual devices. If not specified, no leakage is assumed.
- Return type:
Returns: list of the processed device input information.
- Raises:
ValueError – If the number of impinging_signals does not match the number of registered devices.
- propagate(transmissions, device_states=None, channel_realizations=None, interpolation_mode=InterpolationMode.NEAREST)[source]¶
Propagate device transmissions over the scenario’s channel instances.
- Parameters:
transmissions (Sequence[DeviceOutput]) – Sequence of device transmissisons.
device_states (Sequence[SimulatedDeviceState], optional) – Sequence of device states at the time of signal propagation. If not specified, the device states are assumed to be at the initial state.
channel_realizations (Sequence[ChannelRealization], optional) – Sequence of channel realizations representing the scenario’s channel random states.
interpolation_mode (InterpolationMode, optional) – Interpolation mode for the channel samples. Defaults to InterpolationMode.NEAREST.
- Return type:
- Returns:
Matrix of signal propagations between devices.
list of lists of unique channel realizations linking the devices.
- Raises:
ValueError – If the length of transmissions does not match the number of registered devices.
- realize_channels()[source]¶
Realize all channel instances within the scenario.
Returns: A sequence of channel realizations.
- Return type:
- realize_triggers(devices=None)[source]¶
Realize the trigger models of all registered devices.
Devices sharing trigger models will be triggered simulatenously.
- Parameters:
devices (Sequence[SimulatedDevice], optional) – The devices for which to realize the trigger models. If not specified, all registered devices are considered.
- Return type:
Returns: A sequence of trigger model realizations.
- receive_devices(impinging_signals, states=None, notify=True, trigger_realizations=None, leaking_signals=None)[source]¶
Receive over all simulated scenario devices.
Internally calls
SimulationScenario.process_inputs()
andScenario.receive_operators()
.- Parameters:
impinging_signals (list[Union[DeviceInput, Signal, Iterable[Signal]]]) – List of signals impinging onto the devices.
states (Sequence[SimulatedDeviceState | None], optional) – Sequence of simulated device states at the time of signal impingement. If not specified, the device states are assumed to be at the initial state.
notify (bool, optional) – Notify the receiving DSP layer’s callbacks about the reception results. Enabled by default.
trigger_realizations (Sequence[TriggerRealization], optional) – Sequence of trigger realizations. If not specified, ideal triggerings are assumed for all devices.
leaking_signals (Sequence[Signal] | Sequence[Sequence[Signal]], optional) – Signals leaking from transmit to receive chains within the individual devices. If not specified, no leakage is assumed.
- Return type:
Returns: list of the processed device input information.
- Raises:
ValueError – If the number of impinging_signals does not match the number of registered devices.
- set_channel(alpha_device, beta_device, channel)[source]¶
Specify a channel within the channel matrix.
- Parameters:
alpha_device (SimulatedDevice) – First device to be linked by channel.
beta_device (SimulatedDevice) – Second device to be linked by channel.
channel (Channel) – The channel instance to link alpha_device and beta_device.
- Raises:
ValueError – If alpha_device or beta_device are not registered with this scenario.
- Return type:
- transmit_devices(states=None, notify=True, trigger_realizations=None)[source]¶
Generated information transmitted by all registered devices.
- Parameters:
states (Sequence[SimulatedDeviceState | None], optional) – States of the transmitting devices. If not specified, the current device states will be queried by calling
Device.state()
.notify (bool, optional) – Notify the transmit DSP layer’s callbacks about the transmission results. Enabled by default.
trigger_realizations (Sequence[TriggerRealization], optional) – Realizations of the device’s trigger models. If not spcified, new trigger realizations will be generated from all devices.
- Return type:
Returns: List of generated information transmitted by each device.
- property channels: list[Channel]¶
Unique channel model instances interconnecting devices within this scenario.
- property noise_level: NoiseLevel | None¶
Global noise level of the scenario.
If not set, i.e. None, the noise level is device-specific.
- property noise_model: NoiseModel | None¶
Global noise model of the scenario.
If not set, i.e. None, the noise model is device-specific.
- property visualize: _ScenarioVisualizer¶
- yaml_tag = 'SimulationScenario'¶