FMCW¶
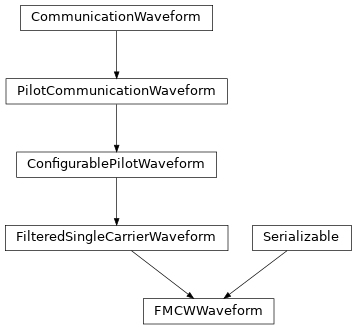
The Frequency-Modulated Continuous Waveform (FMCW) is a single-carrier modulation scheme filtering the communication symbols with rectangle pulse shape:
( ![]() |
( ![]() |
The waveform can be configured by specifying the number of number of data- and preamble symbols contained within each frame, as well as the considered symbol rate:
1# Initialize the waveform description
2waveform = FMCWWaveform(
3 oversampling_factor=128,
4 symbol_rate=1e6,
5 bandwidth=10e6,
6 num_preamble_symbols=16,
7 num_data_symbols=32,
8 modulation_order=64,
9)
Afterwards, additional processing steps such as synchronization, channel estimation, equalization, and the pilot symbol sequence can be added to the waveform:
1# Configure the waveform's synchronization routine
2waveform.synchronization = SingleCarrierCorrelationSynchronization()
3
4# Configure the waveform's channel estimation routine
5waveform.channel_estimation = SingleCarrierLeastSquaresChannelEstimation()
6
7# Configure the waveform's channel equalization routine
8waveform.channel_equalization = SingleCarrierZeroForcingChannelEqualization()
9
10# Configure the waveform's pilot symbol sequence
11waveform.pilot_symbol_sequence = CustomPilotSymbolSequence(
12 np.exp(.25j * np.pi * np.array([0, 1, 2, 3]))
13)
In order to generate and evaluate communication transmissions or receptions, waveforms should be added to modem
implementations.
Refer to Transmitting Modem, Receiving Modem or Simplex Link for more information.
For instructions how to implement custom waveforms, refer to Implementing Communication Waveforms.
- class FMCWWaveform(bandwidth, chirp_duration=0.0, *args, **kwargs)[source]¶
Bases:
FilteredSingleCarrierWaveform
,Serializable
Frequency Modulated Continuous Waveform Filter Modulation Scheme.
- Parameters:
- property bandwidth: float¶
Bandwidth of the frame generated by this generator.
Used to estimate the minimal sampling frequency required to adequately simulate the scenario.
- Returns:
Bandwidth in Hz.
- Return type:
- property chirp_duration: float¶
FMCW Chirp duration.
A duration of zero will result in the inverse symbol rate as chirp duration.
- Returns:
Chirp duration in seconds.
- Raises:
ValueError – If the duration is smaller than zero.
- property chirp_slope: float¶
Chirp slope.
The slope is equal to the chirp bandwidth divided by its duration.
- Returns:
Slope in Hz/s.
- yaml_tag: Optional[str] = 'SC-FMCW'¶
YAML serialization tag