Section¶
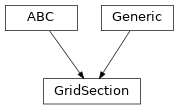
- class GridSection(num_repetitions=1, sample_offset=0, wave=None)[source]¶
-
Description of a part of a grid’s time domain.
- Parameters:
- abstract pick_samples(signal)[source]¶
Pick this section’s samples from the time-domain signal.
- Parameters:
signal (numpy.ndarray) – Time-domain signal to be picked from. Numpy vector of size num_samples.
- Return type:
Returns: Time-domain signal with the section’s samples picked.
- pick_symbols(grid)[source]¶
Pick this section’s data symbols from the resource grid.
- Parameters:
grid (numpy.ndarray) – Resource grid. Two dimensional numpy array of size num_words`x`num_subcarriers.
- Return type:
Returns: Data symbols. Numpy vector of size num_symbols.
- abstract place_samples(signal)[source]¶
Place this section’s samples into the time-domain signal.
- Parameters:
signal (numpy.ndarray) – Time-domain signal to be placed. Numpy vector of size num_samples.
- Return type:
Returns: Time-domain signal with the section’s samples placed.
- place_symbols(data_symbols, reference_symbols)[source]¶
Place this section’s symbols into the resource grid.
- Parameters:
data_symbols (numpy.ndarray) – Data symbols to be placed. Numpy vector of size num_symbols.
reference_symbols (numpy.ndarray) – Reference symbols to be placed. Numpy vector of size num_references.
- Return type:
Returns: Two dimensional numpy array of size num_words`x`num_subcarriers.
- property num_references: int¶
Number of data symbols this section can modulate.
- Returns:
The number of symbols
- Return type:
- property num_repetitions: int¶
Number of section repetitions in the time-domain of an OFDM grid.
- Returns:
The number of repetitions.
- Return type:
- abstract property num_samples: int¶
Number of samples within this OFDM time-section.
- Returns:
Number of samples
- Return type:
- property num_subcarriers: int¶
Number of subcarriers this section requires.
- Returns:
The number of subcarriers.
- Return type:
- property num_symbols: int¶
Number of data symbols this section can modulate.
- Returns:
The number of symbols
- Return type:
- property num_words: int¶
Number of OFDM symbols, i.e. words of subcarrier symbols this section can modulate.
- Returns:
The number of words.
- Return type: