Waveform¶
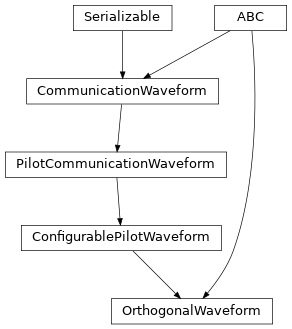
- class OrthogonalWaveform(num_subcarriers, grid_resources, grid_structure, pilot_section=None, pilot_sequence=None, repeat_pilot_sequence=True, **kwargs)[source]¶
Bases:
ConfigurablePilotWaveform
,ABC
Base class for wavforms with orthogonal subcarrier modulation.
- Parameters:
num_subcarriers (int) – Number of available orthogonal subcarriers per symbol.
grid_resources (Sequence[GridResource]) – Grid resources available for modulation.
grid_structure (Sequence[GridSection]) – Grid structure of the time-domain.
pilot_section (PilotSection, optional) – Pilot section transmitted at the beginning of each frame.
pilot_sequence (PilotSymbolSequence, optional) – Sequence of pilot / reference symbols.
repeat_pilot_sequence (bool, optional) – Repeat the pilot sequence if it is shorter than the frame.
- demodulate(signal)[source]¶
Demodulate a base-band signal stream to data symbols.
- Parameters:
signal (np.ndarray) – Vector of complex-valued base-band samples of a single communication frame.
- Return type:
- Returns:
The demodulated communication symbols
- map(data_bits)[source]¶
Map a stream of bits to data symbols.
- Parameters:
data_bits (np.ndarray) – Vector containing a sequence of L hard data bits to be mapped onto data symbols.
- Returns:
Mapped data symbols.
- Return type:
- modulate(symbols)[source]¶
Modulate a stream of data symbols to a base-band signal containing a single data frame.
- Parameters:
data_symbols (Symbols) – Singular stream of data symbols to be modulated by this waveform.
- Return type:
Returns: Samples of the modulated base-band signal.
- pick(placed_symbols)[source]¶
Pick the mapped symbols from the communicaton frame.
Additionally removes interleaved pilot symbols.
- Parameters:
placed_symbols (StatedSymbols) – The placed symbols.
- Return type:
Returns: The symbols with the mapped symbols picked from the frame.
- place(symbols)[source]¶
Place the mapped symbols within the communicaton frame.
Additionally interleaves pilot symbols.
Returns: The symbols with the mapped symbols placed within the frame.
- unmap(symbols)[source]¶
Map a stream of data symbols to data bits.
- Parameters:
symbols (Symbols) – Sequence of K data symbols to be mapped onto bit sequences.
- Returns:
Vector containing the resulting sequence of L data bits In general, L is greater or equal to K.
- Return type:
np.ndarray
- property bit_energy: float¶
Returns the theoretical average (discrete-time) bit energy of the modulated baseband_signal.
Energy of baseband_signal \(x[k]\) is defined as \(\sum{|x[k]}^2\) Only data bits are considered, i.e., reference, guard intervals are ignored.
- property grid_resources: Sequence[GridResource]¶
Available resources within the time-subcarrier grid.
- property grid_structure: Sequence[GridSection]¶
Structure of the time-subcarrier grid.
- property mapping: PskQamMapping¶
Constellation mapping used to translate bit sequences into complex symbols.
- property modulation_order: int¶
Access the modulation order.
- Returns:
The modulation order.
- Return type:
- property num_data_symbols: int¶
Number of bit-mapped symbols contained within each communication frame.
- property num_subcarriers: int¶
Number of available orthogonal subcarriers.
- Raises:
ValueError – If smaller than one.
- property pilot_section: PilotSection | None¶
Static pilot section transmitted at the beginning of each OFDM frame.
Required for time-domain synchronization and equalization of carrier frequency offsets.
- Returns:
FrameSection of the pilot symbols, None if no pilot is configured.
- property pilot_signal: Signal¶
Model of the pilot sequence within this communication waveform.
- Returns:
The pilot sequence.
- Return type:
- property plot_grid: GridVisualization¶
Visualize the resource grid.
- property power: float¶
Returns the theoretical average symbol (unitless) power,
Power of baseband_signal \(x[k]\) is defined as \(\sum_{k=1}^N{|x[k]|}^2 / N\) Power is the average power of the data part of the transmitted frame, i.e., bit energy x raw bit rate
- property symbol_energy: float¶
The theoretical average symbol (discrete-time) energy of the modulated baseband_signal.
Energy of baseband_signal \(x[k]\) is defined as \(\sum{|x[k]}^2\) Only data bits are considered, i.e., reference, guard intervals are ignored.
- Returns:
The average symbol energy in UNIT.