Antenna Arrays¶
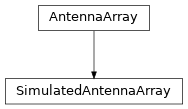
Simulated antenna arrays are the simulation module’s extension of
the core AnteannaArray
decsription by RfChain
models
and polarimetric antenna descriptions.
They are used to configure the frontend description of
SimulatedDevices
.
The current implementation supports two types of antenna arrays:
Array Model |
Description |
---|---|
Custom antenna array with aribtray antenna types, positions and orientations |
|
Uniformly antenna array with equidistantly spaced antennas of the same type |
They can be configured by assigning them to the antennas
attribute
of a SimulatedDevice
:
1simulation = Simulation()
2device = simulation.new_device(carrier_frequency=1e8)
3device.antennas = SimulatedAntennaArray()
The SimulatedAntennaArray in this example snippet has to be replaced by one of the
available implementations.
During simulation runtime, the aforementioned antennas
property can be exploited to compare the performance of different antenna array models:
1uniform_array = SimulatedUniformArray(SimulatedIdealAntenna, 1e-2, [2, 2, 1])
2
3custom_array = SimulatedCustomArray()
4for x, y in np.ndindex((2, 2)):
5 custom_array.add_antenna(SimulatedIdealAntenna(
6 pose=Transformation.From_Translation(np.array([x*1e-2, y*1e-2, 0])),
7 ))
8
9simulation.new_dimension('antennas', [uniform_array, custom_array], device)
In this case, every configured performance evaluator wille be executed for both antenna array models, collecting evaluation samples for each of them.
- class SimulatedAntennaArray(pose=None)[source]¶
Bases:
AntennaArray
[SimulatedAntennaPort
,SimulatedAntenna
]Array of simulated antennas.
- Parameters:
pose (Transformation, optional) – The antenna array’s position and orientation with respect to its device. If not specified, the same orientation and position as the device is assumed.
- analog_digital_conversion(rf_signal, default_rf_chain, frame_duration)[source]¶
Model analog-digital conversion during reception.
- Parameters:
- Return type:
Returns: The base-band digital signal model after analog-digital conversion.
- antenna_state(base_pose)[source]¶
Return the antenna array’s state with respect to a base pose.
- Parameters:
base_pose (Transformation) – The base pose to be used as reference.
- Return type:
Returns: The antenna array’s state with respect to the base pose.
- receive(impinging_signal, default_rf_chain, leaking_signal=None, coupling_model=None)[source]¶
Receive a signal over the antenna array.
- Parameters:
impinging_signal (Signal) – The signal model iminging onto the antenna array over the air.
default_rf_chain (RfChain) – The default RF chain to be used if no RF chain is specified for a port.
leaking_signal (Signal, optional) – The signal model leaking from the antenna array’s transmit chains. If not specified, no leakage is assumed.
coupling_model (Coupling, optional) – The coupling model to be used to simulate mutual coupling between the antenna elements. If not specified, no mutual coupling is assumed.
- Return type:
Returns: The base-band digital signal model after analog-digital conversion.
- rf_chain_modified()[source]¶
Notify the antenna array that the RF chain configuration of one of its antennas has changed.
Automatically called when the
rf_chain
attribute of aSimulatedAntennaPort
a is modified.- Return type:
- transmit(signal, default_rf_chain)[source]¶
Transmit a signal over the antenna array.
The transmission may be distorted by the antennas impulse response / frequency characteristics, as well as by the RF chains connected to the array’s ports.
- Parameters:
- Return type:
Returns: The actually transmitted (distorted) signal model.
- Raises:
ValueError – If the number of signal streams does not match the number of transmit ports.