Pilot Section¶
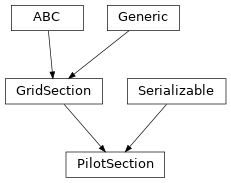
- class PilotSection(pilot_elements=None, wave=None)[source]¶
Bases:
Generic
[OWT
],GridSection
[OWT
],Serializable
Pilot symbol section within an resource grid.
- Parameters:
pilot_elements (Symbols, optional) – Symbols with which the subcarriers within the pilot will be modulated. By default, a pseudo-random sequence from the frame mapping will be generated.
wave (OWT, optional) – The waveform configuration this pilot section is associated with.
- classmethod from_yaml(constructor, node)[source]¶
Recall a new serializable class instance from YAML.
- Parameters:
constructor (SafeConstructor) – A handle to the constructor extracting the YAML information.
node (Node) – YAML node representing the PilotSection serialization.
- Return type:
Returns: The de-serialized object.
- pick_samples(signal)[source]¶
Pick this section’s samples from the time-domain signal.
- Parameters:
signal (np.ndarray) – Time-domain signal to be picked from. Numpy vector of size num_samples.
- Return type:
Returns: Time-domain signal with the section’s samples picked.
- place_samples(signal)[source]¶
Place this section’s samples into the time-domain signal.
- Parameters:
signal (np.ndarray) – Time-domain signal to be placed. Numpy vector of size num_samples.
- Return type:
Returns: Time-domain signal with the section’s samples placed.
- place_symbols(data_symbols, reference_symbols)[source]¶
Place this section’s symbols into the resource grid.
- Parameters:
data_symbols (np.ndarray) – Data symbols to be placed. Numpy vector of size num_symbols.
reference_symbols (np.ndarray) – Reference symbols to be placed. Numpy vector of size num_references.
- Return type:
Returns: Two dimensional numpy array of size num_words`x`num_subcarriers.
- classmethod to_yaml(representer, node)[source]¶
Serialize a serializable object to YAML.
- Parameters:
representer (SafeRepresenter) – A handle to a representer used to generate valid YAML code. The representer gets passed down the serialization tree to each node.
node (PilotSection) – The channel instance to be serialized.
- Return type:
MappingNode
Returns: The serialized YAML node.
- property num_references: int¶
Number of data symbols this section can modulate.
- Returns:
The number of symbols
- Return type:
- property num_repetitions: int¶
Number of section repetitions in the time-domain of an OFDM grid.
- Returns:
The number of repetitions.
- Return type:
- property num_samples: int¶
Number of samples within this OFDM time-section.
- Returns:
Number of samples
- Return type:
- property num_subcarriers: int¶
Number of subcarriers this section requires.
- Returns:
The number of subcarriers.
- Return type:
- property num_symbols: int¶
Number of data symbols this section can modulate.
- Returns:
The number of symbols
- Return type:
- property num_words: int¶
Number of OFDM symbols, i.e. words of subcarrier symbols this section can modulate.
- Returns:
The number of words.
- Return type:
- property pilot_elements: Symbols | None¶
Symbols with which the orthogonal subcarriers within the pilot will be modulated.
- Returns:
A stream of symbols. None, if no pilot symbols were specified.
- Raises:
ValueError – If the configured symbols contains multiple streams.
- property sample_offset: int¶
Offset in samples to the start of the section.
This can be used to explot cyclic prefixes and suffixes in order to be more robust against timing offsets.
- yaml_tag: Optional[str] = 'Pilot'¶
YAML serialization tag