Devices¶
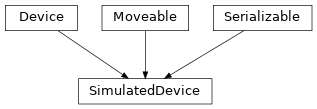
SimulatedDevices
are the description of any entity
capable of transmitting or receiving electromagnetic waves within a HermesPy simulation scenario.
A simulation scenario may feature one or multiple devices freely exchanging electromagnetic waves at
any different carrier frequencies and bandwidths.
Creating a new simulated device is as simple as initializing a new class instance,
or calling new_device
on a Simulation
instance.
1# Create a new stand-alone simulated device
2device = SimulatedDevice()
3
4# Create a new device within a simulation context
5simulation = Simulation()
6device = simulation.new_device()
There exist a number of attributes that can be configured to describe the device’s physical properties, such as its antenna front-end, its radio-frequency chain, the isolation / leakage between transmit and receive chains, the mutual coupling between multiple antennas, the synchronizatio and the hardware noise.
1# Configure the default carrier frequency at wich waveforms are emitted
2device.carrier_frequency = 3.7e9
3
4# Configure the antenna frontend
5device.antennas = SimulatedUniformArray(SimulatedIdealAntenna, .5 * device.wavelength, [4, 4, 1])
6
7# Configure the default rf-chain model
8device.rf_chain = RfChain()
9
10# Configure a transmit-receive isolation model
11device.isolation = SpecificIsolation(
12 1e-10 * np.ones((device.num_receive_antennas, device.num_transmit_antennas))
13)
14
15# Configure a mutual coupling model
16device.coupling = PerfectCoupling()
17
18# Configure a trigger model for synchronization
19device.trigger_model = RandomTrigger()
20
21# Specify a default sampling rate / bandwidth
22device.sampling_rate = 100e6
23
24# Configure a hardware noise model
25device.noise_model = AWGN()
26device.noise_level = N0(dB(-20))
Additionally, within the context of a simulation scenario, users may configure the device’s position, orientation and velocity.
1# Specify the device's postion and orientation in space
2device.position = np.array([10., 10., 0.], dtype=np.float_)
3device.orientation = np.array([0, .125 * np.pi, 0], dtype=np.float_)
4
5# Specify the device's velocity
6device.velocity = np.array([1., 1., 0.], dtype=np.float_)
Note that these properties are only considered by spatial channel models linking to the respective devices, and are otherwise ignored by the simulation engine.
By themselves, devices neither generate any waveforms, nor perform any signal processing on
received waveforms.
Instead, transmit and receive signal processing algorithms have to be assigned to the device’s respective
transmitters
and receivers
slots.
1# Transmit random white noise from the device
2transmitter = SignalTransmitter(Signal.Create(
3 np.random.normal(size=(device.num_transmit_ports, 100)) +
4 1j * np.random.normal(size=(device.num_transmit_ports, 100)),
5 device.sampling_rate,
6 device.carrier_frequency
7))
8device.transmitters.add(transmitter)
9transmitter.device = device # Equivalent to the previous line
10
11# Receive a signal without additional processing at the device
12receiver = SignalReceiver(100, device.sampling_rate, expected_power=1.)
13device.receivers.add(receiver)
14receiver.device = device # Equivalent to the previous line
The selected singnal processing algorithms in the snippet above are rather simplistic and only generate a static signal that is continuously transmitted, and receive a fixed number of samples, respectively. More complex algorithms implementing communication modems, radars or joint communication and sensing algorithms are available in HermesPy’s Communication, Sensing and JCAS modules.
Outside of full-fledged Monte Carlo simulations, users may inspect the output of a configured simulated device
by calling the transmit
method.
1# Generate a transmission from the device
2transmission = device.transmit()
Similarly, users may inspect the signal processing result of configured receive signal processing algorithms
by calling the receive
method
and providing a Signal
model of the assumed waveform impinging onto the device.
1# Receive a signal at the device
2impinging_signal = Signal.Create(
3 np.random.normal(size=(device.num_transmit_ports, 100)) +
4 1j * np.random.normal(size=(device.num_transmit_ports, 100)),
5 device.sampling_rate,
6 device.carrier_frequency
7)
8reception = device.receive(impinging_signal)
The transmit
routine is a wrapper around multiple subroutines,
that are individually executed during simulation runtime for performance optimization reasons, finally returning a
SimulatedDeviceTransmission
dataclass containing
all information generated during the simulation of the device’s transmit chain.
The receive
method requires the input of the signal to be processed by the device,
and is also a wrapper around multiple subroutines, that are individually executed during simulation runtime for performance optimization reasons,
finally returning a SimulatedDeviceReception
dataclass containing
all information generated during the simulation of the device’s receive chain.
- class SimulatedDevice(scenario=None, antennas=None, rf_chain=None, isolation=None, coupling=None, trigger_model=None, sampling_rate=None, carrier_frequency=0.0, noise_level=None, noise_model=None, pose=None, velocity=None, power=1.0, seed=None)[source]¶
Bases:
Device
,Moveable
,Serializable
Representation of an entity capable of emitting and receiving electromagnetic waves.
A simulation scenario consists of a collection of devices, interconnected by a network of channel models.
- Parameters:
scenario (Scenario, optional) – Scenario this device is attached to. By default, the device is considered floating.
antennas (SimulatedAntennaArray, optional) – Antenna array model of the device. By default, a single ideal istropic antenna is assumed.
rf_chain (RfChain, optional) – Model of the device’s radio frequency amplification chain. If not specified, a chain with ideal hardware models will be assumed.
isolation (Isolation, optional) – Model of the device’s transmit-receive isolations. By default, perfect isolation is assumed.
coupling (Coupling, optional) – Model of the device’s antenna array mutual coupling. By default, ideal coupling behaviour is assumed.
trigger_model (TriggerModel, optional) – The assumed trigger model. By default, a
StaticTrigger
is assumed.sampling_rate (float, optional) – Sampling rate at which this device operates. By default, the sampling rate of the first operator is assumed.
carrier_frequency (float, optional) – Center frequency of the mixed signal in rf-band in Hz. Zero by default.
snr (float, optional) – Signal-to-noise ratio of the device. By default, the device is assumed to be noiseless.
snr_type (SNRType, optional) – Type of the signal-to-noise ratio. By default, the signal-to-noise ratio is specified in terms of the power of the noise to the expected power of the received signal.
pose (Transformation | Trajectory, optional) – Position and orientation of the device in time and space. If a Transformation is provided, the device is assumed to be static. If not specified, the device is assumed to be at the origin with zero velocity.
velocity (np.ndarray, optional) – Initial velocity of the moveable in local coordinates. By default, the moveable is assumed to be resting. Only considered if pose is a Transformation, otherwise the velocity is assumed from the Trajectory.
power (float, optional) – Power of the device. Assumed to be 1.0 by default.
seed (int, optional) – Seed of the device’s pseudo-random number generator.
- generate_output(operator_transmissions=None, cache=True, trigger_realization=None)[source]¶
Generate the simulated device’s output.
- Parameters:
operator_transmissions (List[Transmissions], optional) – List of operator transmissions from which to generate the output. If the operator_transmissions are not provided, the transmitter’s caches will be queried.
cache (bool, optional) – Cache the generated output at this device. Enabled by default.
trigger_realization (TriggerRealization, optional) – Trigger realization modeling the time delay between a drop start and frame start. Perfect triggering is assumed by default.
- Return type:
Returns: The device’s output.
- Raises:
RuntimeError – If no operator_transmissions were provided and an operator has no cached transmission.
- process_from_realization(impinging_signals, realization, trigger_realization=None, leaking_signal=None, cache=True)[source]¶
Simulate a signal reception for this device model.
- Parameters:
impinging_signals (DeviceInput | Signal | Sequence[Signal] | SimulatedDeviceOutput) – List of signal models arriving at the device. May also be a two-dimensional numpy object array where the first dimension indicates the link and the second dimension contains the transmitted signal as the first element and the link channel as the second element.
realization (SimulatedDeviceRealization) – Random realization of the device reception process.
trigger_realization (TriggerRealization, optional) – Trigger realization modeling the time delay between a drop start and frame start. Perfect triggering is assumed by default.
leaking_signal (Signal, optional) – Signal leaking from transmit to receive chains. If not specified, no leakage is considered during signal reception.
cache (bool, optional) – Cache the resulting device reception and operator inputs. Enabled by default.
- Return type:
Returns: The received information.
- Raises:
ValueError – If device_signals is constructed improperly.
- process_input(impinging_signals, cache=True, trigger_realization=None, noise_level=None, noise_model=None, leaking_signal=None)[source]¶
Process input signals at this device.
- Parameters:
impinging_signals (DeviceInput | Signal | Sequence[Signal] | SimulatedDeviceOutput) – List of signal models arriving at the device. May also be a two-dimensional numpy object array where the first dimension indicates the link and the second dimension contains the transmitted signal as the first element and the link channel as the second element.
cache (bool, optional) – Cache the resulting device reception and operator inputs. Enabled by default.
trigger_realization (TriggerRealization, optional) – Trigger realization modeling the time delay between a drop start and frame start. Perfect triggering is assumed by default.
noise_level (NoiseLevel, optional) – Level of the simulated hardware noise. If not specified, the device’s configured noise level will be assumed.
noise_model (NoiseModel, optional) – Model of the simulated hardware noise. If not specified, the device’s configured noise model will be assumed.
leaking_signal (Signal, optional) – Signal leaking from transmit to receive chains.
- Return type:
Returns: The processed device input.
- realize_reception(noise_level=None, noise_model=None, cache=True)[source]¶
Generate a random realization for receiving over the simulated device.
- Parameters:
noise_level (NoiseLevel, optional) – Level of the simulated hardware noise. If not specified, the device’s configured noise level will be assumed.
noise_model (NoiseModel, optional) – Model of the simulated hardware noise. If not specified, the device’s configured noise model will be assumed.
cache (bool, optional) – Cache the generated realization at this device. Enabled by default.
- Return type:
Returns: The generated realization.
- receive(impinging_signals, cache=True, trigger_realization=None)[source]¶
Receive information at this device.
- Parameters:
impinging_signals (DeviceInput | Signal | Sequence[Signal] | SimulatedDeviceOutput) – List of signal models arriving at the device. May also be a two-dimensional numpy object array where the first dimension indicates the link and the second dimension contains the transmitted signal as the first element and the link channel as the second element.
cache (bool, optional) – Cache the resulting device reception and operator inputs. Enabled by default.
trigger_realization (TriggerRealization, optional) – Trigger realization modeling the time delay between a drop start and frame start. Perfect triggering is assumed by default.
- Return type:
Returns: The processed device input.
- state(timestamp=0.0)[source]¶
Immutable physical state of the device during simulation drop generation.
- Parameters:
timestamp (float, optional) – Global timestamp in seconds. By default, zero is assumed.
- Return type:
DeviceState
Returns: Device state at the given timestamp.
- transmit(cache=True, trigger_realization=None)[source]¶
Transmit over this device.
- Parameters:
cache (bool, optional) – Cache the transmitted information. Enabled by default.
trigger_realization (TriggerRealization, optional) – Trigger realization modeling the time delay between a drop start and frame start. Perfect triggering is assumed by default.
- Return type:
Returns: Information transmitted by this device.
- property antennas: SimulatedAntennaArray¶
Antenna array model of the simulated device.
- property attached: bool¶
Attachment state of this device.
- Returns:
True if the device is currently attached, False otherwise.
- Return type:
- property carrier_frequency: float¶
Central frequency of the device’s emissions in the RF-band.
- Returns:
Carrier frequency in Hz.
- Return type:
frequency (float)
- Raises:
ValueError – On negative carrier frequencies.
- property coupling: Coupling¶
Model of the device’s antenna array mutual coupling behaviour.
Returns: Handle to the coupling model.
- property input: ProcessedSimulatedDeviceInput | None¶
Most recent input of this device.
Updated during
receive()
andreceive_from_realization()
.- Returns:
The input information. None if
receive()
orreceive_from_realization()
has not been called yet.
- property isolation: Isolation¶
Model of the device’s transmit-receive isolation.
Returns: Handle to the isolation model.
- property noise: float¶
Shorthand property for accessing the noise level of the device.
- Raises:
ValueError – For negative noise levels.
- property noise_level: NoiseLevel¶
Level of the simulated hardware noise.
- property noise_model: NoiseModel¶
Model of the simulated hardware noise.
- property operator_separation: bool¶
Separate operators during signal modeling.
- Returns:
Enabled flag.
- property output: SimulatedDeviceOutput | None¶
Most recent output of this device.
Updated during
transmit()
.- Returns:
The output information. None if
transmit()
has not been called yet.
- property realization: SimulatedDeviceReceiveRealization | None¶
Most recent random realization of a receive process.
Updated during
realize_reception()
.- Returns:
The realization. None if
realize_reception()
has not been called yet.
- rf_chain: RfChain¶
Model of the device’s radio-frequency chain.
- property sampling_rate: float¶
Sampling rate at which the device’s analog-to-digital converters operate.
- Returns:
Sampling rate in Hz. If no operator has been specified and the sampling rate was not set, a sampling rate of \(1\) Hz will be assumed by default.
- Raises:
ValueError – If the sampling rate is not greater than zero.
- property scenario: Scenario | None¶
Scenario this device is attached to.
- Returns:
Handle to the scenario this device is attached to. None if the device is considered floating.
- serialized_attribute = {'adc', 'rf_chain'}¶
- property trigger_model: TriggerModel¶
The device’s trigger model.