Animation¶
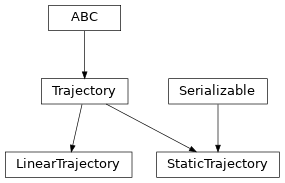
Movements of devices and other objects within simulations may be described by Trajectories
,
which model the veloctiy, position and orientation of the object as a function of time.
Generally, objects that may be animated within a simulation should inherit from the Moveable
class,
which exposes the trajectory
property.
The currently available trajectory types are:
Type |
Description |
---|---|
A trajectory that does not change over time. |
|
A trajectory that moves in a straight line at a constant velocity. |
The following example demonstrates how to assign a linear trajectory to a device within a simulation and plot the scenario:
1# Create a new simulation featuring two devices
2simulation = Simulation()
3device_alpha = simulation.new_device()
4device_beta = simulation.new_device()
5
6# Assign each device a linear trajectory
7device_alpha.trajectory = LinearTrajectory(
8 initial_pose=Transformation.From_Translation(np.array([0, 0, 20])),
9 final_pose=Transformation.From_Translation(np.array([0, 100, 5])),
10 duration=60,
11)
12device_beta.trajectory = LinearTrajectory(
13 initial_pose=Transformation.From_Translation(np.array([100, 100, 0])),
14 final_pose=Transformation.From_Translation(np.array([0, 0, 0])),
15 duration=60,
16)
17
18# Visualize the trajectories
19simulation.scenario.visualize()
20plt.show()
- class Trajectory[source]¶
Bases:
ABC
Base class for motion trajectories of moveable objects within simulation scenarios.
- class StaticTrajectory(pose=None, velocity=None)[source]¶
Bases:
Serializable
,Trajectory
A helper class generating a static trajectory.
- sample(timestamp)[source]¶
Sample the trajectory at a given point in time.
- Parameters:
timestamp (float) – Time at which to sample the trajectory in seconds.
- Return type:
Returns: A sample of the trajectory.
- property max_timestamp: float¶
Maximum timestamp of the trajectory in seconds.
For times greater than this value the represented object’s pose is assumed to be constant.
- property pose: Transformation¶
Static pose of the object.
- class LinearTrajectory(initial_pose, final_pose, duration, start=0.0)[source]¶
Bases:
Trajectory
A helper class generating a linear trajectory between two poses.
- class Moveable(trajectory)[source]¶
Bases:
object
Base class of moveable objects within simulation scenarios.
- Parameters:
trajectory (Trajectory, optional) – Trajectory this object is following. If not provided, the object is assumed to be static.
- property trajectory: Trajectory¶
Motion trajectory this object is following.
- class TrajectorySample(timestamp, pose, velocity)[source]¶
Bases:
object
Dataclass for a single pose sample within a trajectory.
- Parameters:
timestamp (float) – Time at which the trajectory was sampled in seconds.
pose (Timestamp) – Pose of the object at the given time.
velocity (np.ndarray) – Velocity of the object at the given time.
- property pose: Transformation¶
Pose of the object at the given time.