OCDM¶
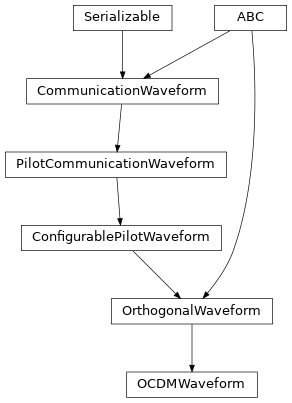
Orthogonal Chirp Division Multiplexing (OCDM) is a method of encoding digital data into multiple orthogonal chirps, i.e. waveforms with zero cross-correlation.
Considering a simplex-link scenario of two modems communicating over a 3GPP 5G TDL channel
1# Initialize a simulation with two dedicated devices for transmission and reception
2carrier_frequency = 3.7e9
3simulation = Simulation()
4tx_device = simulation.new_device(carrier_frequency=carrier_frequency)
5rx_device = simulation.new_device(carrier_frequency=carrier_frequency)
6
7# Assume a 5G TDL channel model
8channel = TDL(TDLType.A, 1e-7, doppler_frequency=10)
9simulation.set_channel(tx_device, rx_device, channel)
10
11# Link the devices
12link = SimplexLink(tx_device, rx_device)
configuring an OCDM waveform requires the specification of the resource-time grid onto which the transmitted data and pilot symbols are placed:
1# Configure an orthogonal waveform featuring 128 subcarriers
2grid_resources = [
3 GridResource(16, PrefixType.CYCLIC, .1, [GridElement(ElementType.DATA, 7), GridElement(ElementType.REFERENCE, 1)]),
4 GridResource(128, PrefixType.CYCLIC, .1, [GridElement(ElementType.DATA, 1)]),
5]
6grid_structure = [
7 SymbolSection(64, [0, 1])
8]
9waveform = OCDMWaveform(
10 bandwidth=128e3,
11 grid_resources=grid_resources,
12 grid_structure=grid_structure,
13 num_subcarriers=128,
14)
15link.waveform = waveform
The grid considers \(128\) orthogonal subcarriers each modulated with a unique symbol, with \(128\) repetitions in time-domain, so that overall \(16384\) symbols are transmitted per frame. The grid alternates between two types of symbol sections, one carrying a reference element on every \(8\)-th subcarrier and one consisting only of data symbols.
Additionally, post-processing routines for channel estimation and channel equalization may be specified on the waveform level
1# Configure channel estimation and equalization
2waveform.channel_estimation = OrthogonalLeastSquaresChannelEstimation()
3waveform.channel_equalization = ZeroForcingChannelEqualization()
4
5# Configure frame synchronization
6waveform.pilot_section = PilotSection()
7waveform.synchronization = CorrelationSynchronization()
- class OCDMWaveform(bandwidth, num_subcarriers, grid_resources, grid_structure, pilot_section=None, pilot_sequence=None, repeat_pilot_sequence=True, **kwargs)[source]¶
Bases:
OrthogonalWaveform
Orthogonal Chirp Division Multiplexing waveform.
- Parameters:
num_subcarriers (int) – Number of available orthogonal subcarriers per symbol.
grid_resources (Sequence[GridResource]) – Grid resources available for modulation.
grid_structure (Sequence[GridSection]) – Grid structure of the time-domain.
pilot_section (PilotSection, optional) – Pilot section transmitted at the beginning of each frame.
pilot_sequence (PilotSymbolSequence, optional) – Sequence of pilot / reference symbols.
repeat_pilot_sequence (bool, optional) – Repeat the pilot sequence if it is shorter than the frame.